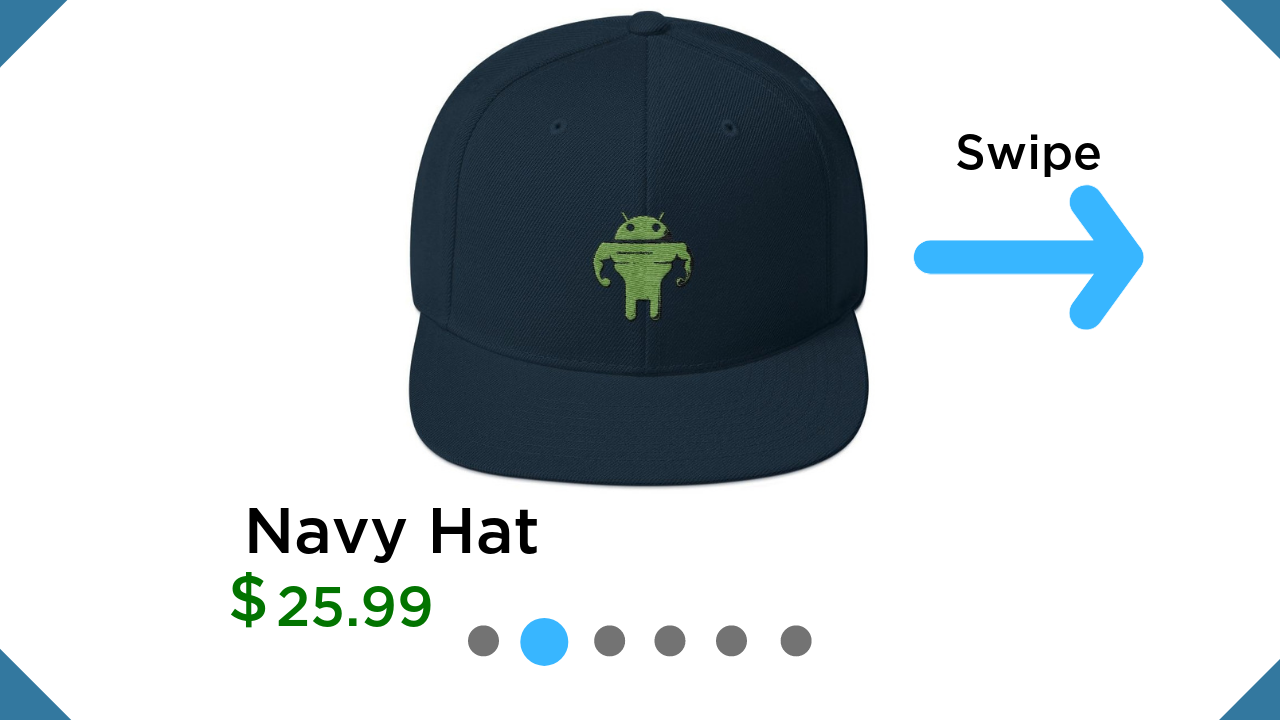
Example:
Table of Contents:
- Introduction
- Dependencies
- Drawable Resources
- colors.xml
- Modeling the Data
- Resource Class
- Pager Adapter
- Tab Selectors
- The Layouts
- Big Decimal Utility Class
- ViewPager Item Fragment
- MainActivity
- Final Thoughts
Prefer to watch instead of read? Click here
Introduction
In Android apps it's common practice to give users the ability to swipe through product variations. A good example of this is different colors of the same product.
In the example above I have a ViewPager with 6 different fragments added to it. Below the ViewPager is a TabLayout. When the user is viewing a particular tab, the dot is blue. When they are not viewing a tab the dot is grey.
Dependencies
- Glide is needed for displaying the images.
- The design support library is needed to use a TabLayout.
Drawable Resources
Download
Click the "Download" button above and download all the drawable resources required for this example. Download them all and place them into your projects drawables folder.
Colors
Modeling the Data
When building any application it's always a good idea to prepare the data models first. That way you can get a good idea for what the views need to look like.
In this example I'm displaying different colored hats. So I'll built a "Hat" POJO.
Parcelable
Notice that the POJO is Parcelable. It must be parcelable so it can be attached to a bundle. You'll see this in action later on.BigDecimal
Also notice that the price field is a BigDecimal type. You can read more about why I'm using BigDecimal to display currency here.Resource Class
This class is for preparing the resources that will be displayed in the ViewPager. There's 6 different colors.
Pager Adapter
The first step in implementing a ViewPager is creating an adapter class. You have two choices when it comes to an adapter:
-
FragmentPagerAdapter:
A FragmentPagerAdapter is best for use when there are a handful of typically more static fragments to be paged through. The fragment of each page the user visits will be kept in memory.
-
FragmentStatePagerAdapter:
This version of the pager is more useful when there are a large number of pages, working more like a list view. When pages are not visible to the user, their entire fragment may be destroyed, only keeping the saved state of that fragment.
The FragmentStatePagerAdapter is best for paging across a collection of objects for which the number of pages is undetermined. Since we could technically have any number of product variations, this is the best choice.
Tab Selectors
To set the different states of the TabLayout you need to use something known as a "selector". The TabLayout has two possible states (see image below). Selected and unselected. If it's unselected, the dot is grey. If it's selected, the dot is blue.
To set these two states I need to set a special xml attribute to the TabLayout. Here are the files that I'll use when setting that attribute. They belong in the drawables directory.
The Layouts
We need two layout files for this example.
-
One for MainActivity which will contain the TabLayout and ViewPager.
Notice the "tabBackground" attribute. That is the selectors.
-
One for ViewPagerItemFragment which is the fragment class that holds each item in the ViewPager. NOTE: I haven't built this class yet. It's two sections below.
Big Decimal Utility Class
We need a class that can convert the price field to the correct number of decimals. This class will achieve that.
ViewPager Item Fragment
Each item in the ViewPager is a fragment. A fragment that contains a different product variation. This is the fragment class that will display each of those items.
NOTE: To attach the Hat objects to a bundle they must be Parcelable. See the Hat class above for a Parcelable implementation.
MainActivity
And finally we have MainActivity. MainActivity is where the ViewPager and TabLayout live. In the init() method, I'm looping through all the hat variations in the Hats class and instantiating a new fragment for each one. Each Hat object is attached as an argument to the fragment.
Final Thoughts
Implementing a system like this is a great way to swipe through images that are related. Another example of how you might use this is swiping through pages of an E-Book or large document. You could use the ViewPager to display the different pages of a large text.
Staying in the Loop
If you want to stay in the loop and get an email when I write new blog posts, Follow me on Instagram or join the CodingWithMitch community on my website. It only takes about 30 seconds to register.
Authors
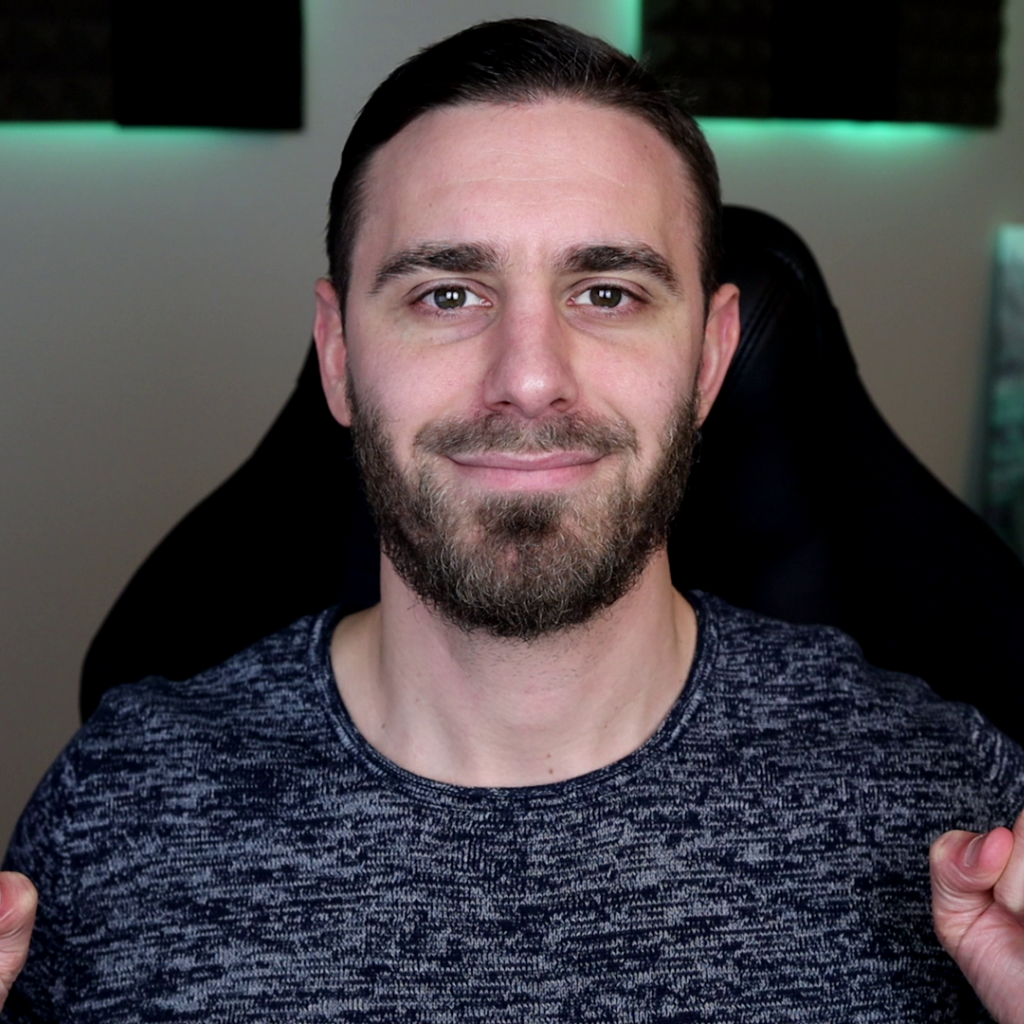
Create an Account
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
Comments