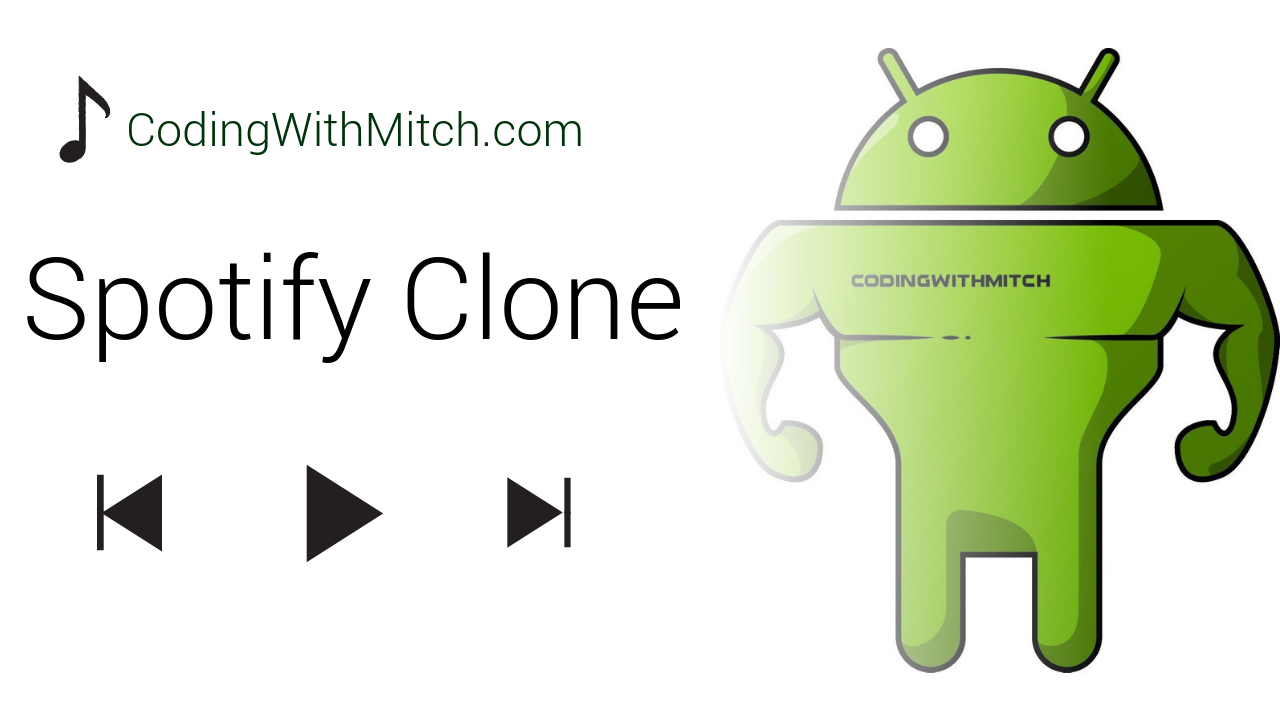
Audio Streaming on Android
Watch demoDescription
Build an audio streaming application that looks similar to Spotify.
In this course I teach you the current best practice way to build an audio streaming application on android. To make it more interesting, I designed it to look similar to the popular music streaming app called Spotify.
I use the ExoPlayer library for streaming the audio files. The audio files are located online in a Firebase Firestore database.
Here's what you will see in the course:
- Every line of code is written and explained on video
- ExoPlayer (same media library used by YouTube in the mobile app)
- Streaming audio files from the internet (Firestore)
- Playlists
- Effective fragment management
- AsyncTasks
- SharedPreferences
- Effective communication with interfaces
- Services (a class specifically built to stream media files)
- Background Services that run forever
- Notifications
- Controlling playback through a notification
- Notifications and the lock screen
- Broadcast Receivers
- Glide library to download bitmaps
- RecyclerViews
- And much more...
Take this journey with me and learn the best practice way to build an audio streaming application on Android.
Metadata
Published: Aug. 2, 2021
Lectures: 53
Total video time: 06:59:21
Authors
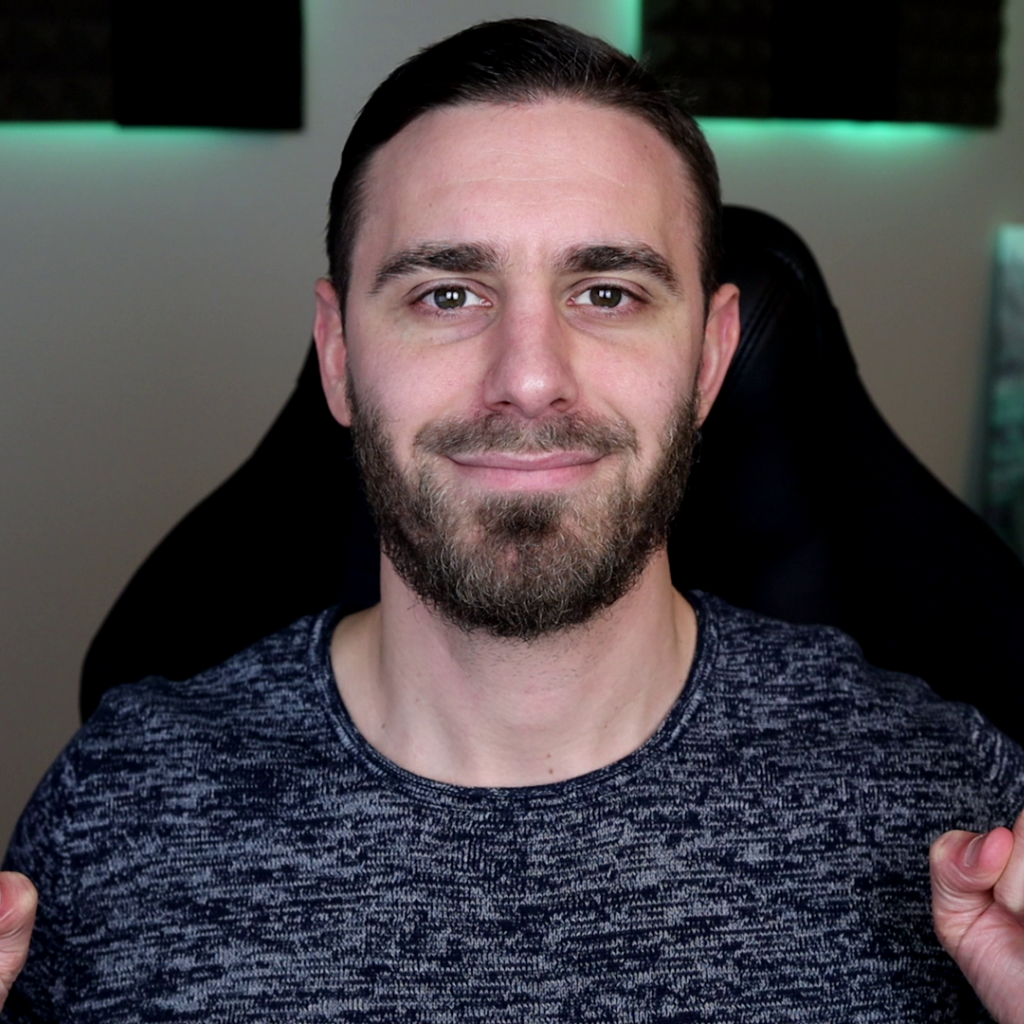
Audio Streaming on Android
If you restart this course, all your progress will be reset
Lectures
-
Course Demo
-
Using the Source Code
-
Dependencies and SDK Versions
-
Retrieving Audio Data from Firestore
-
Building the Layouts
-
fragment_home
-
fragment_media_controller
-
List-item Layouts
-
RecyclerView Adapter for HomeFragment
-
RecyclerView Setup for HomeFragment
-
Retrieving Firestore Data in HomeFragment
-
Improving the Query Experience in HomeFragment
-
RecyclerView Setup for CategoryFragment
-
Retrieving Firestore Data in CategoryFragment
-
Testing Firestore Query in CategoryFragment
-
RecyclerView Setup for PlaylistFragment
-
Retrieving Firestore Data in PlaylistFragment
-
MediaController Setup
-
Implementing Fragment Transactions
-
Custom Fragment Management
-
Preventing Fragments from Stacking
-
Fragment Back Navigation
-
Implementing the Fragment Navigation
-
ActionBar Titles
-
Handling Configuration Changes Part 1
-
Service Class to Stream Audio
-
MediaBrowserServiceCompat Basics
-
Audio Focus and Audio Noisy Intent
-
ExoPlayer Setup Part 1 (Initialization)
-
ExoPlayer Setup Part 2 (playing a media file)
-
ExoPlayer Setup Part 3 (tracking playback state)
-
Associating the ExoPlayer with the Media Service
-
Starting and Binding to the Media Service
-
Playing the First Media Files
-
Dynamic Playlists with an Application Instance
-
Playing a Media Item with OnClick
-
Subscribing to a Playlist
-
Setting the Queue Position
-
Resolving the Playlist Subscription Issue
-
Detecting Changes to the Playback State
-
Updating the UI with Media Information
-
Broadcast Receiver for Seekbar Progress
-
Seeking Playback
-
Updating the UI when Playback is Complete
-
Handling Configuration Changes Part 2
-
Restoring Recently Played Media
-
Notification Overview
-
Notifications on Android Oreo
-
Notification Basics
-
Media Button Receivers and Pending Intents
-
Creating and Destroying a Notification
-
Controlling Playback from a Notification
-
Handling Configuration Changes Part 3
-
Setting a Bitmap in a Notification
Comments
Lectures
-
Course Demo
-
Using the Source Code
-
Dependencies and SDK Versions
-
Retrieving Audio Data from Firestore
-
Building the Layouts
-
fragment_home
-
fragment_media_controller
-
List-item Layouts
-
RecyclerView Adapter for HomeFragment
-
RecyclerView Setup for HomeFragment
-
Retrieving Firestore Data in HomeFragment
-
Improving the Query Experience in HomeFragment
-
RecyclerView Setup for CategoryFragment
-
Retrieving Firestore Data in CategoryFragment
-
Testing Firestore Query in CategoryFragment
-
RecyclerView Setup for PlaylistFragment
-
Retrieving Firestore Data in PlaylistFragment
-
MediaController Setup
-
Implementing Fragment Transactions
-
Custom Fragment Management
-
Preventing Fragments from Stacking
-
Fragment Back Navigation
-
Implementing the Fragment Navigation
-
ActionBar Titles
-
Handling Configuration Changes Part 1
-
Service Class to Stream Audio
-
MediaBrowserServiceCompat Basics
-
Audio Focus and Audio Noisy Intent
-
ExoPlayer Setup Part 1 (Initialization)
-
ExoPlayer Setup Part 2 (playing a media file)
-
ExoPlayer Setup Part 3 (tracking playback state)
-
Associating the ExoPlayer with the Media Service
-
Starting and Binding to the Media Service
-
Playing the First Media Files
-
Dynamic Playlists with an Application Instance
-
Playing a Media Item with OnClick
-
Subscribing to a Playlist
-
Setting the Queue Position
-
Resolving the Playlist Subscription Issue
-
Detecting Changes to the Playback State
-
Updating the UI with Media Information
-
Broadcast Receiver for Seekbar Progress
-
Seeking Playback
-
Updating the UI when Playback is Complete
-
Handling Configuration Changes Part 2
-
Restoring Recently Played Media
-
Notification Overview
-
Notifications on Android Oreo
-
Notification Basics
-
Media Button Receivers and Pending Intents
-
Creating and Destroying a Notification
-
Controlling Playback from a Notification
-
Handling Configuration Changes Part 3
-
Setting a Bitmap in a Notification