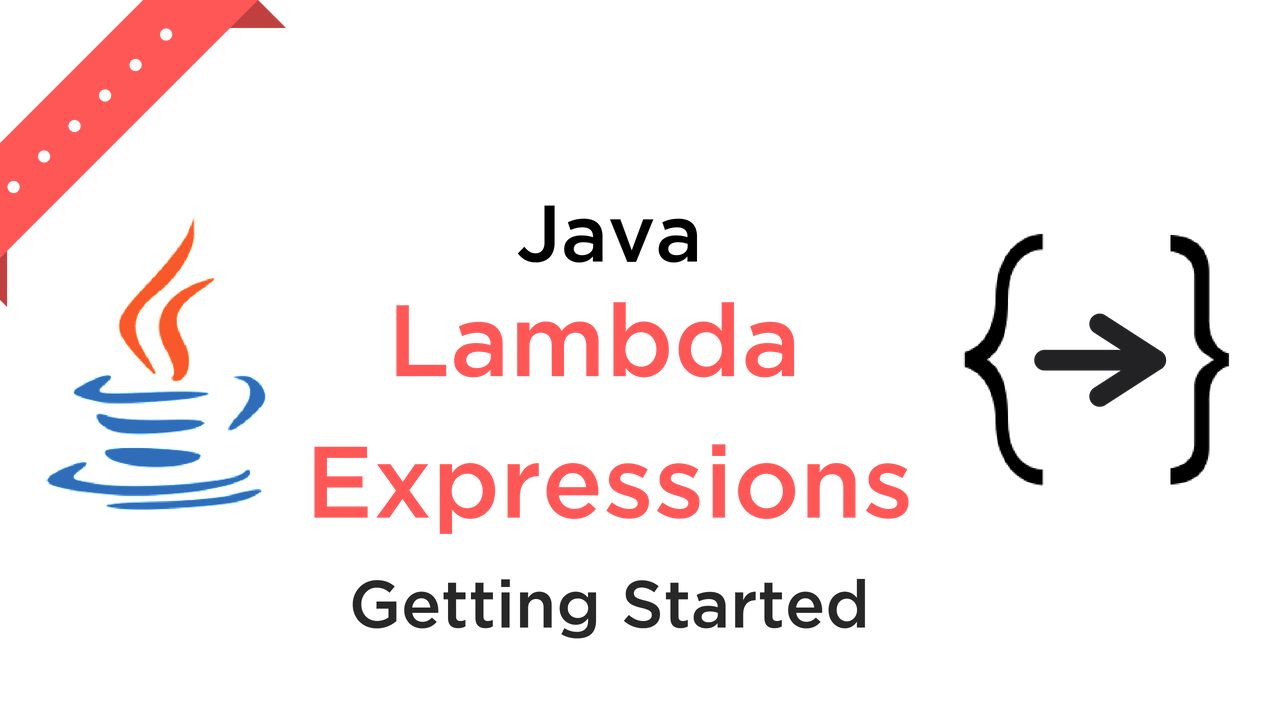
Table of Contents:
Introduction
In this blog I'm going to show you how to work with lambda expressions in Java
What is Lambda Expression?
Java lambda expressions are new in Java 8. Java lambda expressions are Java's first step into functional programming. A Java lambda expression is thus a function which can be created without belonging to any class. A Java lambda expression can be passed around as if it was an object and executed on demand.
Characteristics of Lambdas Expressions
There are some characteristics of Lambda Expressions in Java.
- Curly braces
While using Lambda Expressions in Java, there is no need to use curly braces in expression body if the body contains a single statement. - return keyword
There is no need to use return keyword in expression body if there is single expression in expression body. - Paranthesis
There is no need to enclose the single parameter in paranthesis. But, if there are more than one parameters, then paranthesis are required. - Type declaration
It is optional to declare the data type of the parameters in paranthesis. Compiler will automatically detect it's type using it's value.
Lambda Expressions App
Now, Let's dive into the code. We will build the simple app which will demonstrate some basic examples of Lambda Expressions in Java.
Let's begin the coding!!!
Dependencies
Open build.gradle (Module:app) and add the following dependencies under android {...} section. This will enable to Java 8 support in the app, because Java 8 supports lambda expressions.
Designing Splash Activity Layout
Add the following code in your activity_splash_screen.xml file. This layout contains one TextView which depicts the name of the app Lambda Expressions Example
Setting Up SplashActivity
In this section, we will write the code to display Splash screen for few seconds without using Lambda Expressions as well as by using Lambda Expressions and after that it will go to MainActivity
- Without Lambda Expression :
In this part, we will see how to write the code for Splash Screen without using Lambda Expressions.
Paste the below code in the onCreate() method of SplashScreen Activity. Basically, this is delaying the operation defined inside run() method for specified number of seconds. In this case, we are specifying 2 seconds as the delay time. After 2 seconds, MainActivity will open up.
- With Lambda Expression :
In this part, we will see how to write the code for Splash Screen by using Lambda Expressions.
In the below code, we are using Lambda Expression while writing code for Splash Screen Activity.
When we are calling postDelayed() function, we are passing an anonymous function as it's parameter (It will automatically override run() method of the Runnable interface) followed by -> operator (arrow operator) which is basically used for defining the body of the function.
When there is only one line of statement in the function body, then we can only pass -> operator to define it's body. But, when there are more than one line of statements in the function body, then, we have to define the function body by the following way () -> { } Here, curly braces are added to define the function body.
As you have seen here, Lambda Expressions helps in writing cleaner code that is easily understandable
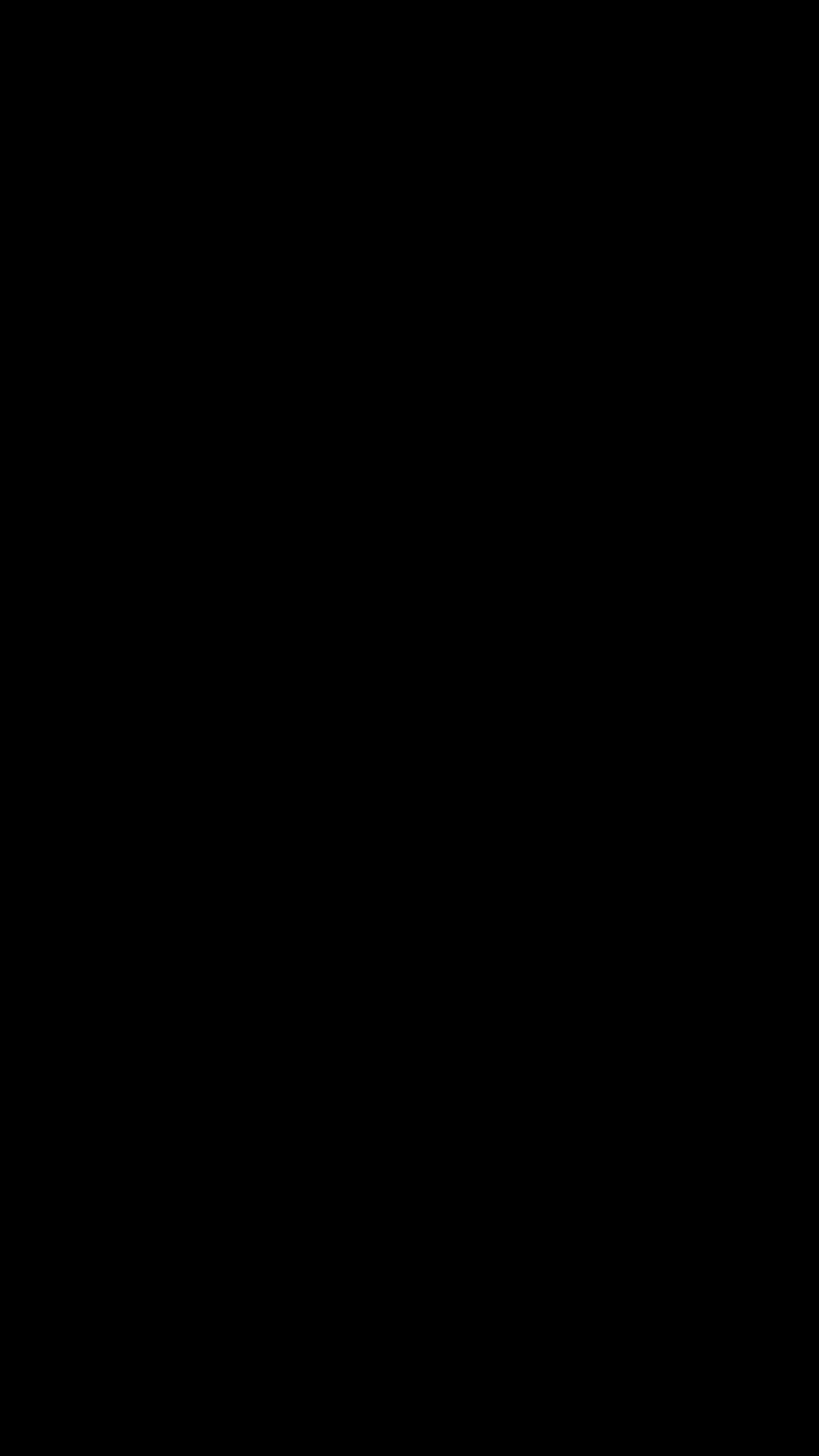
Designing MainActivity Layout
Add the following code in your activity_main.xml file. This layout contains two EditTexts for email and password and two buttons are also added in this layout file to demonstrate an example of Lambda Expressions
Setting Up MainActivity
In this section, we will write some code to show some examples with Lambda Expressions in Java
- Without Lambda Expression :
- First, declare the Button object and find the reference of that button using findViewById() method inside onCreate() method.
- Second, we are just applying the onClickListener on this button without using Lambda Expression, here, we have to explicitly override the onClick() method of the onClickListener interface. But when we use Lambda Expression here, it will not be the case. We will see this example with Lambda Expression in the next part.
- With Lambda Expression :
-
Here, we are calling setOnClickListener() method and as it's parameter, we are passing a view variable which was the parameter of the onClick() method.
Here, we are not defining the type of the view parameter because it's one of the characteristic of Lambda Expression that if there is one parameter then we don't need to define the type of the variable. Compiler will automatically detect it's type.
Finally, we are displaying the Toast inside the function body. Here, the function body is defined followed by the -> operator, because, as we have seen in the previous section, if there is only one statement in the function body then, it can be followed by a -> operator.
-
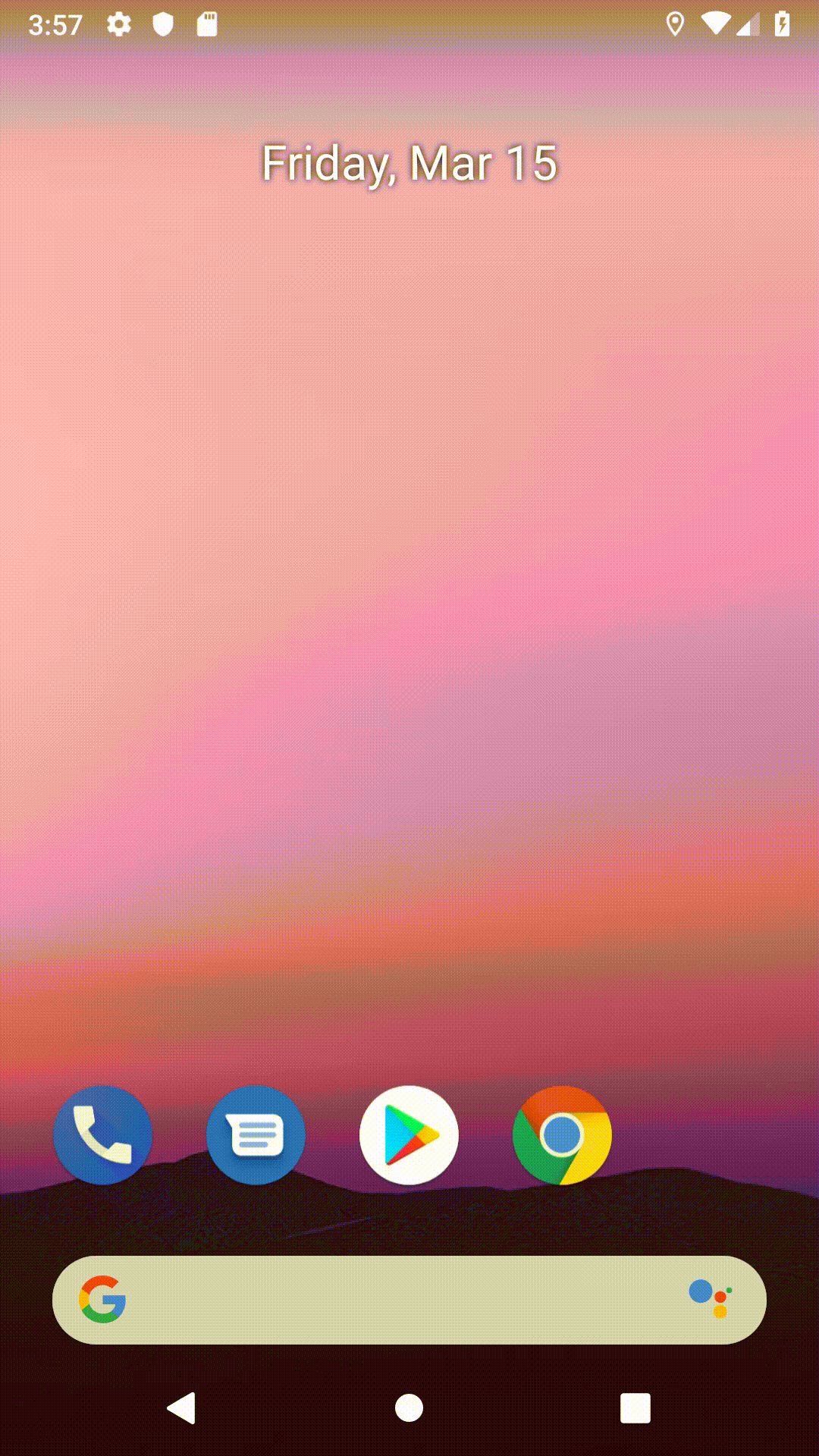
Demonstrating Method Reference
In this section, we will see another feature of Lambda Expression. It's called as Method Reference
Method Reference : Sometimes, however, a lambda expression does nothing but call an existing method. In those cases, it's often clearer to refer to the existing method by name. That's where, Method references comes into play. They enable you to do this; they are compact, easy-to-read lambda expressions for methods that already have a name.
- Without using Method Reference :
- First, declare the Button object and find the reference of that button using findViewById() method inside onCreate() method.
- Second, we are just calling the setOnClickListener() method on the second button. Inside the parameter to it, we are just using a lambda expression and calling the handleClick() method. But, this syntax can be modified into Method Reference. We'll see that in next part.
- With Method Reference :
-
Here, we are calling setOnClickListener() method and as it's parameter, we are passing this::handleClick()
This type of calling of handleClick() method is called as Reference to an instance method. It just means that, here, the lambda expression is doing nothing, it just calles the handleClick() method by it's name.
this::handleClick() statement is basically equivalent to (view) -> handleClick(view) statement.
-
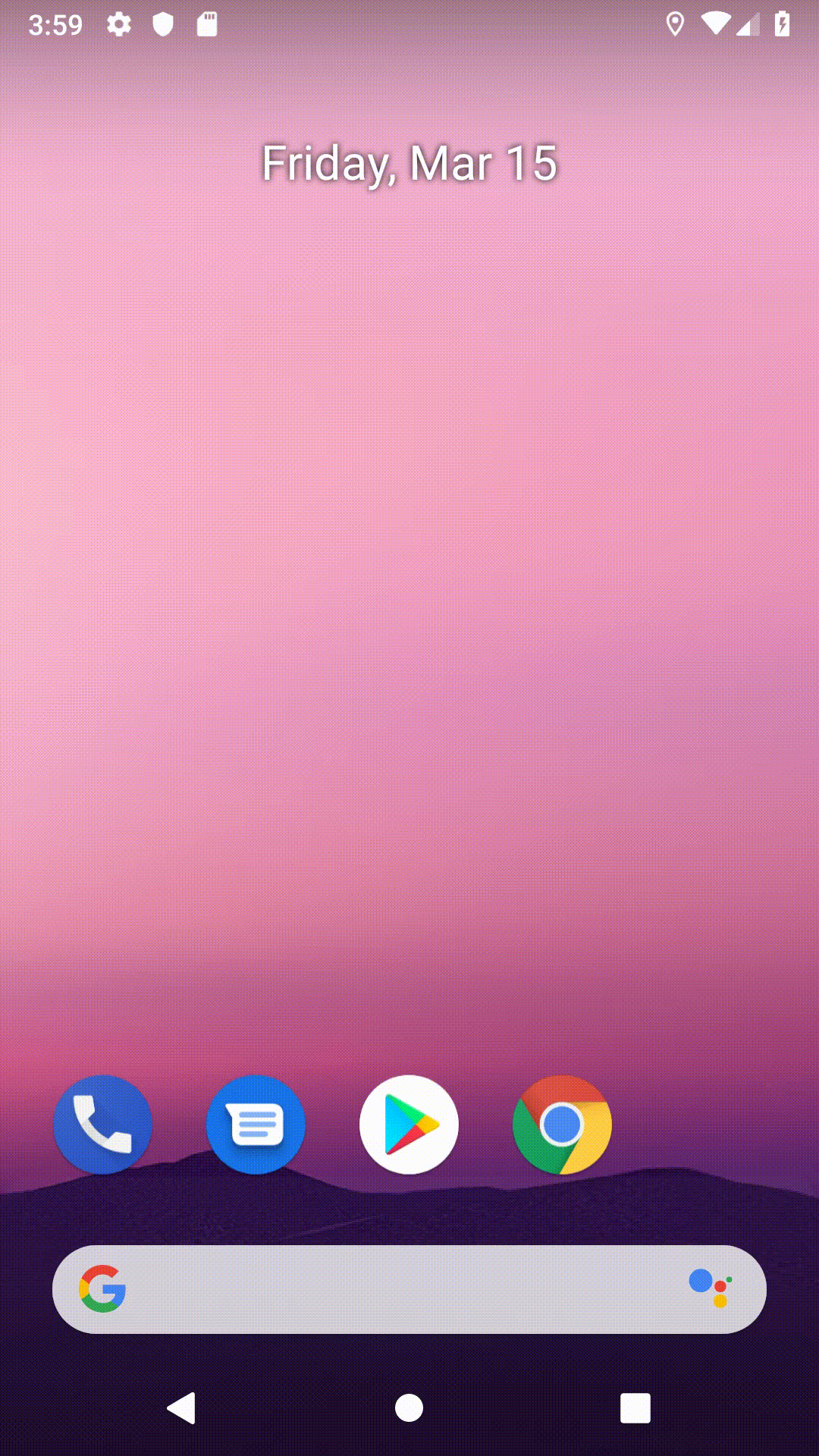
Functional Interface
In this section, we will see another feature of Lambda Expression. It's called as Functional Interface
Functional Interface : An interface with exactly one abstract method is called Functional Interface. @FunctionalInterface annotation is added so that we can mark an interface as functional interface.
- First, declare the two EditTexts object and find the reference of that editTexts using findViewById() method inside onCreate() method.
- Second, create an interface named ToastInterface and mark it with @FunctionalInterface annotation. And create one abstract method inside this interface and name it as createToast(). Pass the two parameters to it, first, email and second, password.
-
First, make an object of ToastInterface and initialize it. In the below code, we are writing toastInterface = (email, password) -> .... This means that, we are anonymously calling the createToast(email, password) method using the Lambda Expression and followed by the -> operator, we are defining it's body (Displaying email id in Toast)
Second, we are calling setOnClickListener() method using Lambda Expression and calling onClick() method anonymously. In this case, we are defining the body of the function using arrow operator followed by curly braces ((view) -> {}). In the function body, we are calling createToast() method of the ToastInterface and passing email and password as it's parameter.
Because it's one of the characteristic of Lambda Expression that, if there are more than one line of statements inside the function body, then, the appropriate way to define the function body is this.
(view -> { "function body" });
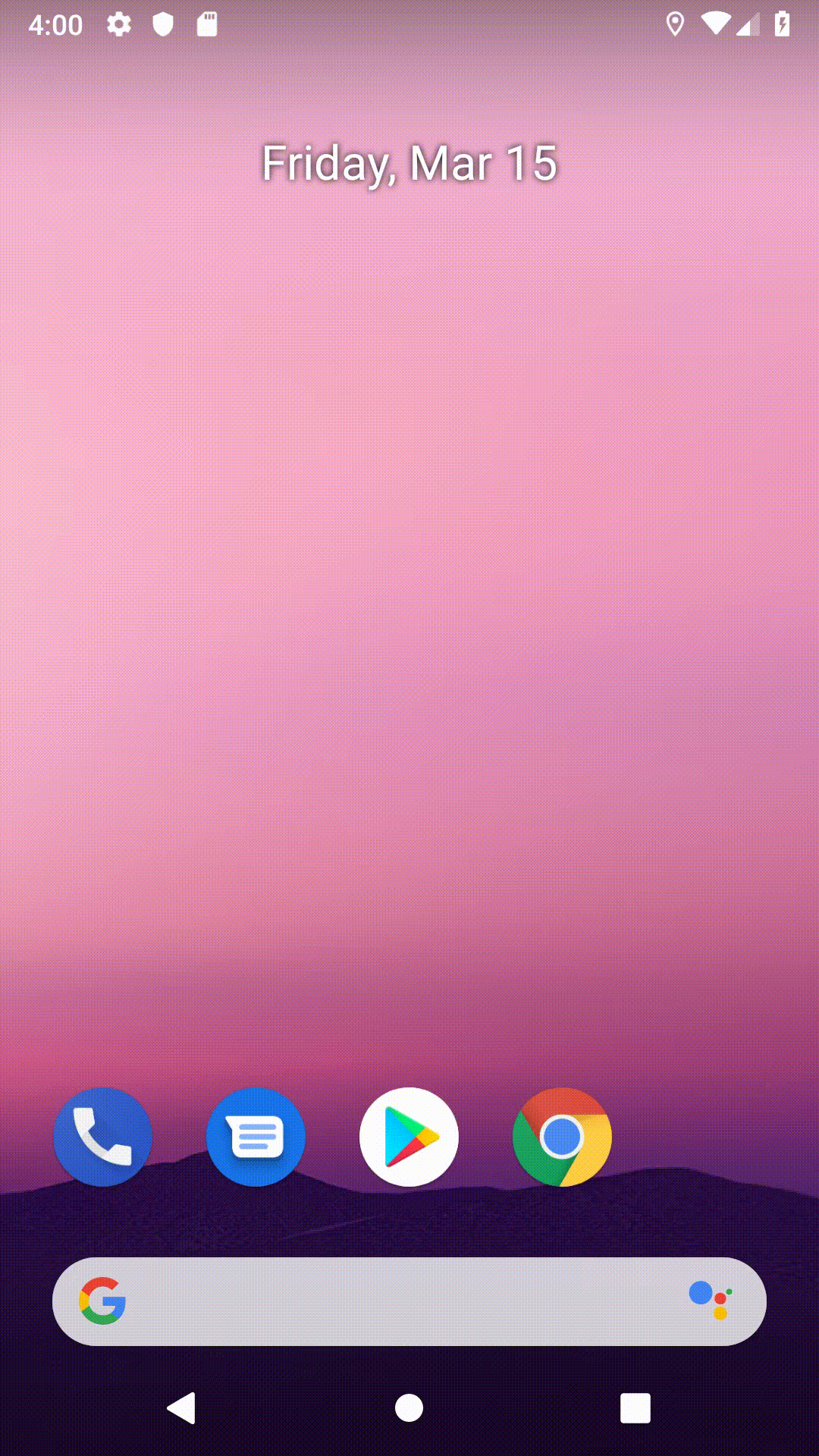
That's All! Happy Coding!
The complete code is available here.
Staying in the Loop
If you want to stay in the loop and get an email when I write new blog posts, Follow me on Instagram or join the CodingWithMitch community on my website. It only takes about 30 seconds to register.
Authors
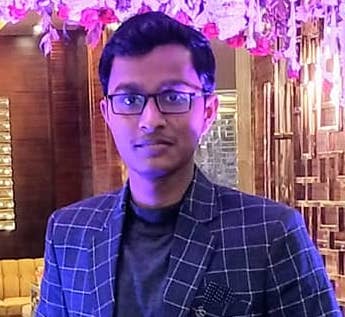
Create an Account
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
Comments