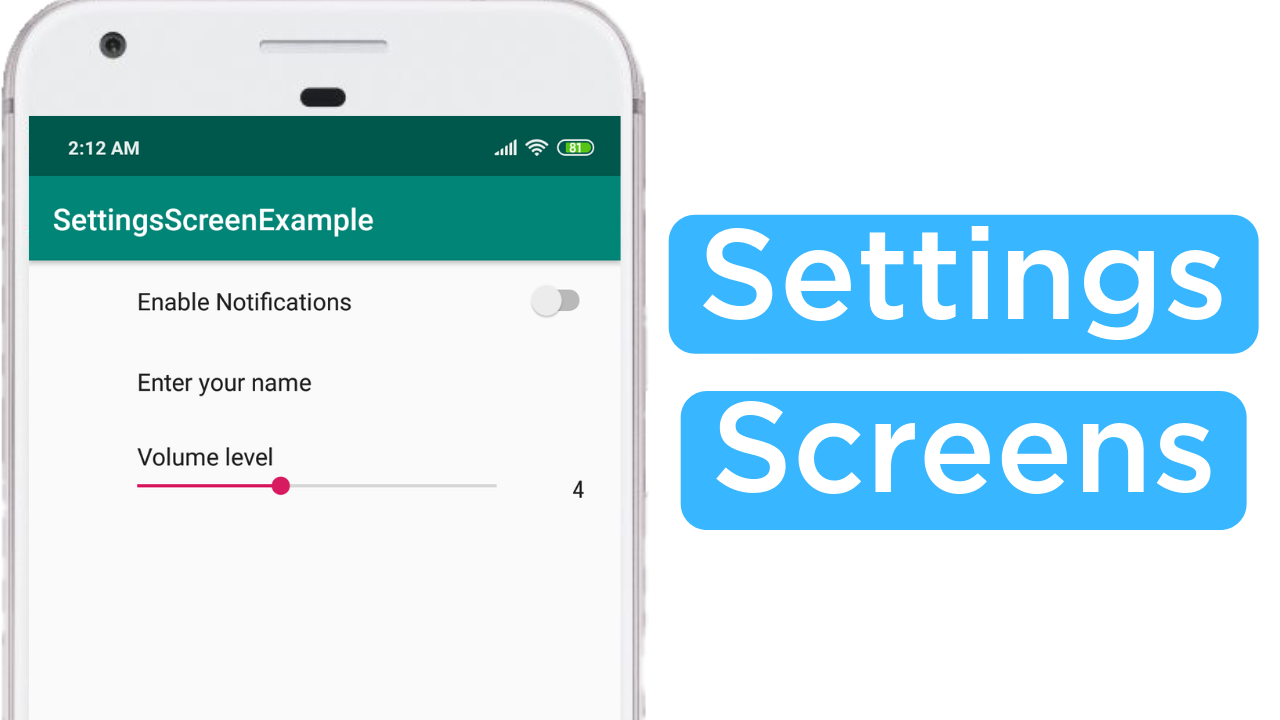
Table of Contents:
- Demo
- Introduction
- What is the Preferences Framework?
- When you should use it?
- When you should not use it?
-
An Example:
Demo
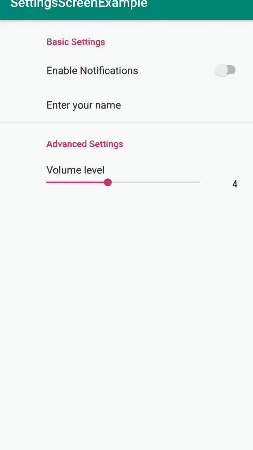
Introduction
In this blog I'm going to show you how to work with Shared Preferences and the Preferences Framework in Android.
What is the Preferences Framework?
Android system provides many ways to save data for your application.One of them is Preferences Framework , through Android preference framework we can easily develop screen that allows user to modify preferences. It takes the value and saves them to internal storage on behalf of your application in the form of key-value pairs and it stores all primitive types(booleans,int,strings etc).
When you should use it?
- When the small amount of data is to be stored:
Shared Preferences can be used to store small amount of data like single text , some numerical value or saving some user settings. - When the length of time saved is more for data:
The data stored in Shared preferences remains in file till the app is uninstalled.It persists across the app restarts and even phone restarts.
When you should not use it?
- When the amount of data is more:
When you want to store large amount of data , Shared preferences is not the option then you should go for local database or cloud database also you should not save login information and anything to do with billing. That means no credit cards or other sensitive information.
Settings Screen Demo
We will build the simple app which contains the settings screen in which we will store different types of data to Shared Preferences.
Let's begin the coding!!!
Dependencies
Open build.gradle (Module:app) and add the following dependency. This dependency is required for "PreferenceFragmentCompat" class.
Layout for Settings Screen
Under "res" folder create new Android resource directory called "xml" and inside that directory create new xml resource file called "preferences.xml" and add the following code , this file contains the layout for the settings screen.
The root node of this file should be "PreferenceScreen" element and inside this tag we have our individual preference. Some of the preferences are listed below.
- SwitchPreference:
This preference uses the switch element and has two states (on/off) , the value stored for this preference is of boolean type. We can set some properties to this preference listed below.
- title: Sets the title for the preference.
- key: Sets the key for the preference , this key is used to retreive the value from Shared preferences.
- defaultValue: Sets the default or initial value to the preference.
- EditTextPreference: This preference uses the EditText element , when this preference is clicked it shows the dialog for user to input a text , the value stored for this preference is of String type.
- SeekBarPreference: This preference uses the Seekbar element and stores the integer value to preferences. We can provide properties like "minValue" , "maxValue" etc.
onSharedPreferenceChanged
This is a callback function which is called when shared preferences is changed , this callback will be run on your main thread.
Note: In onStart() we have to register a sharedPreferencesChangeListener so that it can listen to the changes in SharedPreferences and if any change occur callback function "onSharedPreferenceChanged" will be invoked and in onStop() we have to unregister a listener to avoid memory leaks.
Settings Fragment
Create new blank fragment named "SettingsFragment" and add the following code inside it.
What is PreferenceFragmentCompat?
PreferenceFragmentCompat is an abstract class which extends from class Fragment that shows the hierarchy of preference objects as lists. Advantage of using this class is that it automatically stores these preference to Shared Preferences as the user interacts with them.
In addition to this if we want to retreive the value from SharedPreferences then it can be done by sharedPreferences object which will be instantiated by "getDefaultSharedPreferences()."
What is addPreferencesFromResource() ?
This method loads the preferences from an XML resource file. It takes one argument which is id of XML file to inflate.
SwitchPreference
Open SettingsFragment.java and inside "onSharedPreferenceChanged()" add the following code. Inside this we are checking if the key whose value is changed is equal to "notificationKey" then retreive that value and based on that value display the toast to the user.
EditTextPreference
Open SettingsFragment.java and inside "onSharedPreferenceChanged()" add the following code. Inside this we are checking if the key whose value is changed is equal to "nameKey" then retreive that value and based on that value display the toast to the user.
SeekBarPreference
Open SettingsFragment.java and inside "onSharedPreferenceChanged()" add the following code. Inside this we are checking if the key whose value is changed is equal to "volumeKey" then retreive that value and based on that value display the toast to the user.
Attach Settings Fragment to Activity
Open activity_main.xml and MainActivity.java file and add the following code. In this we are attaching a SettingsFragment to an Activity. That's it.
Creating Setting Groups
Open preferences.xml file and add the following code. In this we are grouping our settings elements this makes easier for user to understand and to not make list seem too overwhelming.
Before Creating Groups
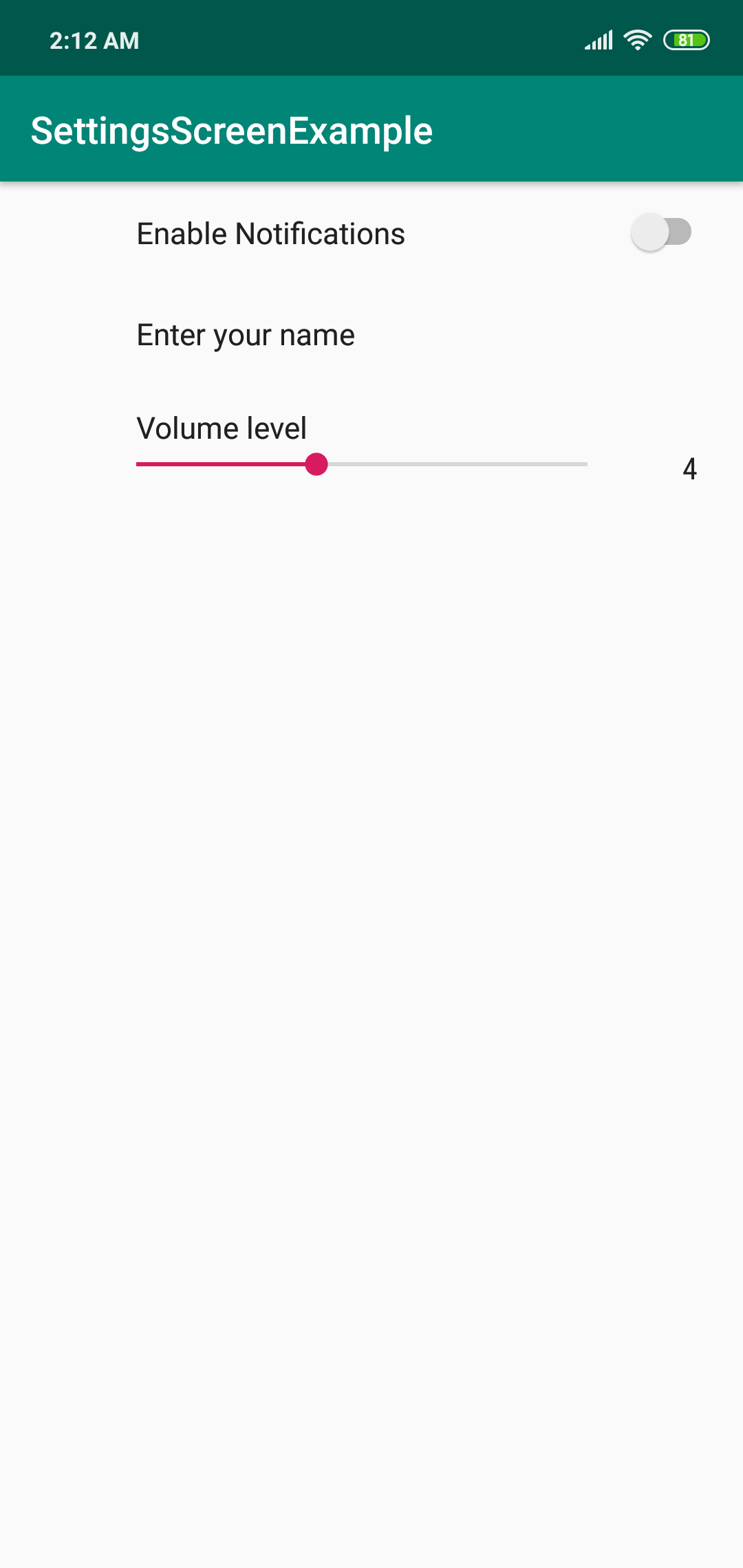
After Creating Groups
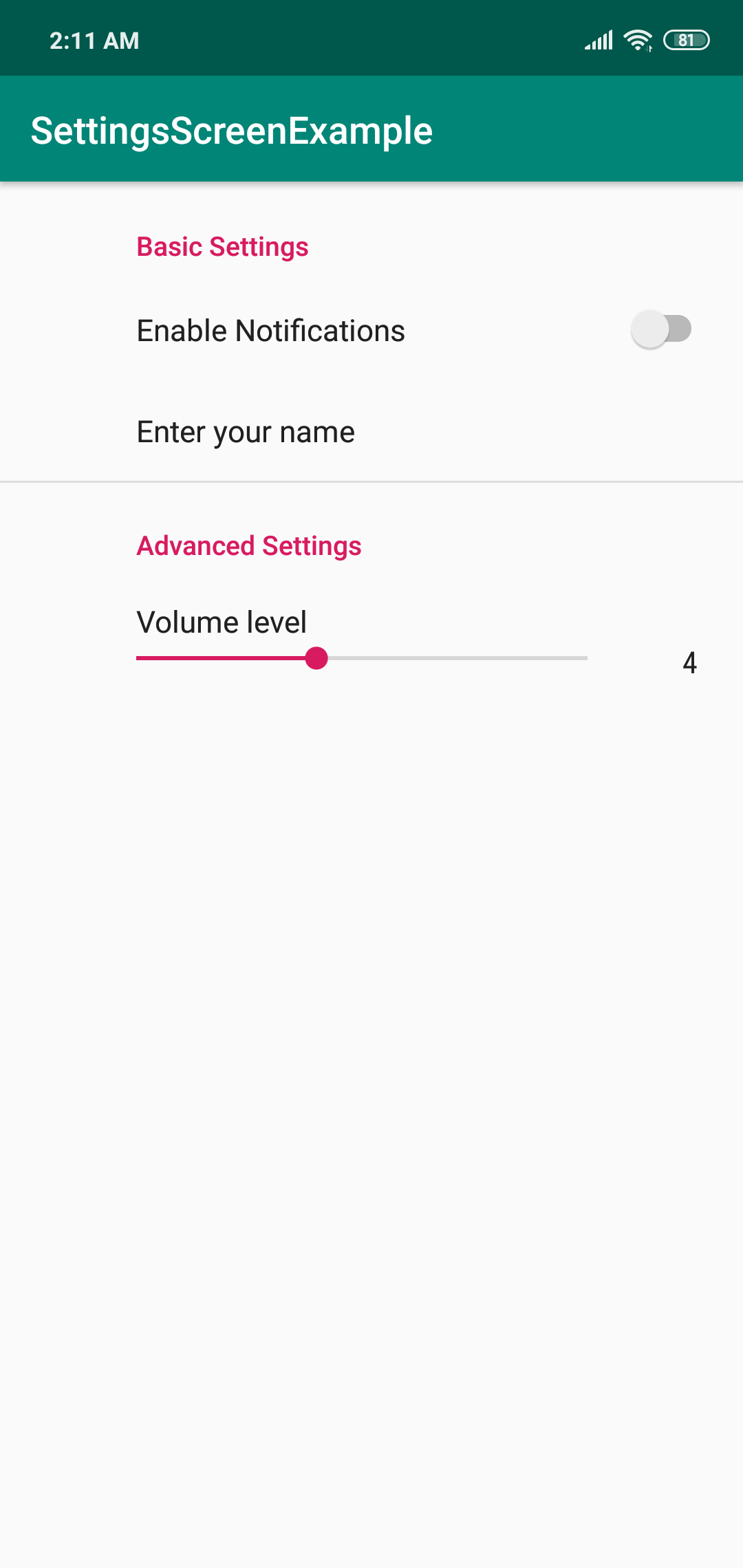
That's All! Happy Coding!
The complete code is available here.
Staying in the Loop
If you want to stay in the loop and get an email when I write new blog posts, Follow me on Instagram or join the CodingWithMitch community on my website. It only takes about 30 seconds to register.
Authors
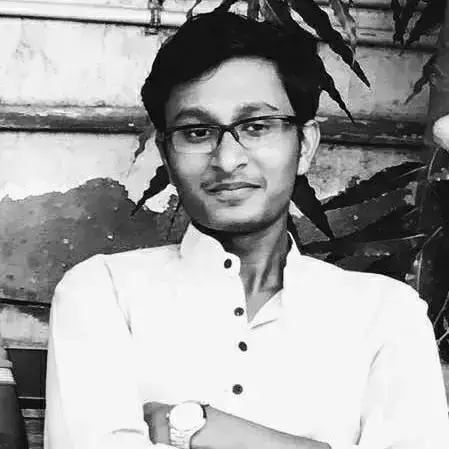
Create an Account
Similar Posts
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
Comments