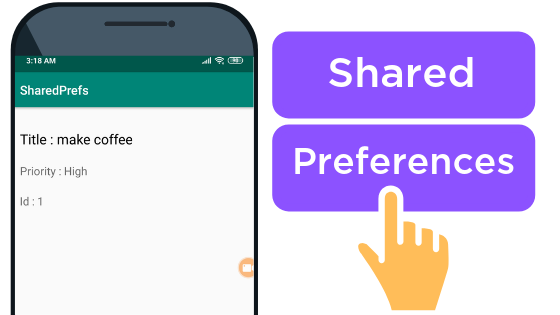
Table of Contents:
- Demo
- Introduction
- What is Shared Preferences?
- When you should use it?
- When you should not use it?
-
An Example:
Demo
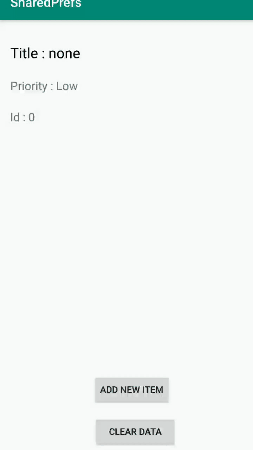
Introduction
In this blog I'm going to show you how to work with Shared Preferences in Android.
What is Shared Preferences?
Android system provides many ways to save data for your application . One of them is Shared Preferences . Shared Preferences allows you to save data in key-value pairs and it stores all primitive types(booleans,int,strings etc).
When you should use it?
- When the small amount of data is to be stored:
Shared Preferences can be used to store small amount of data like single text , some numerical value or saving some user settings. - When the length of time saved is more for data:
The data stored in Shared preferences remains in file till the app is uninstalled . It persists across the app restarts and even phone restarts.
When you should not use it?
- When the amount of data is more:
When you want to store large amount of data , Shared preferences is not the option then you should go for local database or cloud database.
Todo App
We will build the simple Todo App which will store the title , id and priority of TODO in Shared preferences and on other screen , details of TODO will be displayed by retreiving data from preferences.
Note: In this blog we will be storing multiple primitive data types to Shared Preferences such as Boolean , Integer , Strings .
Let's begin the coding!!!
Designing Layout
Open activity_main.xml file and add the following code . It contains three textViews for title , id , priority and one button for "Add new Item" which will navigate to AddTodoActivity, also create another empty activity named AddTodoActivity and open activity_add_todo.xml and add the following code . It contains two Editext for title and id , two Radio buttons for priority and one button for "Add Todo".
Initialization
Open AddTodoActivity.java file and add the following code . We need an Editor to edit and save to preferences . To get access to preferences we need to first initialize the SharedPreferences . This can be done in three ways:- getPreferences() : when you want to get activity specific preferences , this can be used from acitvity.
- getSharedPreferences() : when you want to get application specific preferences , this can be used from activity or other application context.
- getDefaultSharedPreferences() : when you want to get preferences that work with Android's preference framework.
Note: "getDefaultSharedPreferences" will be what you should use 99% of the time . It's the most general.
Note: getSharedPreferences() takes two arguments - prefs file name and operating mode . Some of the operating modes are discussed below:
- MODE_PRIVATE : when you want your file to not access outside your application.
- MODE_APPEND : when you want to append the preferences with the already existing preferences.
- MODE_WORLD_READABLE : when you want all applications to have read access to your file , Deprecated in API level 17.
- MODE_WORLD_WRITEABLE : when you want all applications to have write access to your file , Deprecated in API level 17.
Storing data to Preferences
Open AddTodoActivity.java file and add the following code , on click of "Add Todo" button we are storing title,id and priority to preferences.
Note: When the state of RadioButton changes the function "onRadioButtonClicked" gets called , if RadioButton "High" is checked then priority is set to true else false.
Storing data requires two things - one is key(always a string) and other is value
What is the difference between commit() and apply() ?
- commit() : Writes the data synchronously , blocking the thread its called from . It returns a true value if the operation was successful and false in other case .
- apply() : Writes the data asynchronously , it does not returns anything whether the operation is successful or not .
How to view SharedPreferences file ?
First connect your device then in Android studio go to Device File Explorer => data => data => "package_name" => shared_prefs => your xml file
After storing data , shared preferences file will look like this :
Retreiving data from Preferences
Open MainActivity.java file and add the following code , We are retreiving data from preferences file and displaying it in textViews.
Retreiving data requires two things - one is key(always a string) and other is default value which we provided is based on our datatypes.
Clear Data
Open MainActivity.java file and add the following code . We can clear the data from Shared Preferences by editor.clear() function . In this we have added another button on click of which we cleared the data from TodoPrefs.xml .
That's All! Happy Coding!
The complete code is available here.
Authors
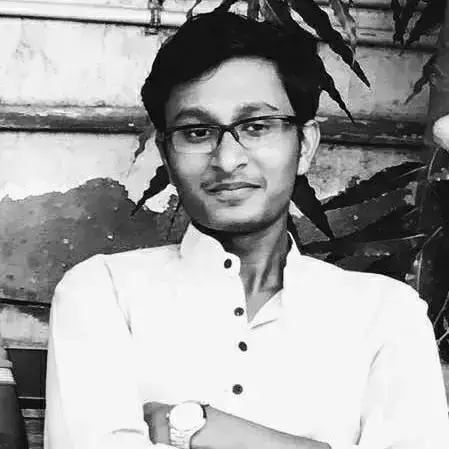
Create an Account
Similar Posts
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
Comments