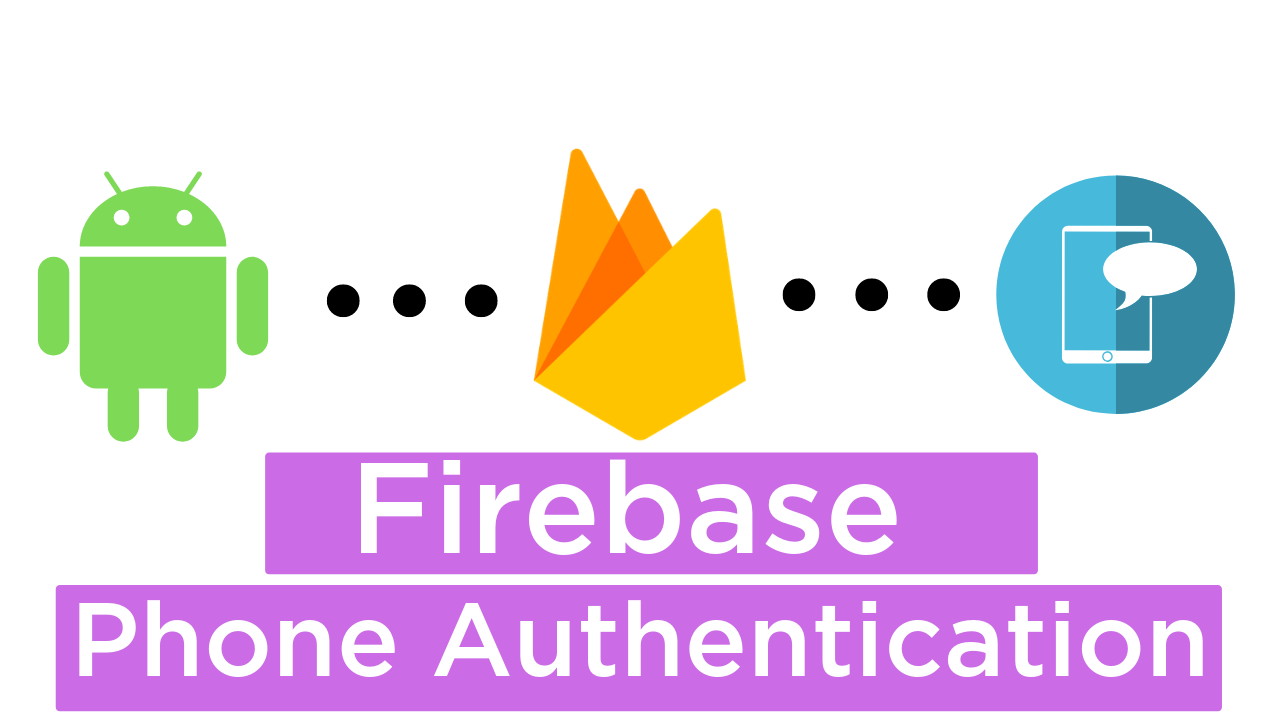
Table of Contents:
- Demo
- Introduction
- What is Firebase ?
-
An Example:
Demo
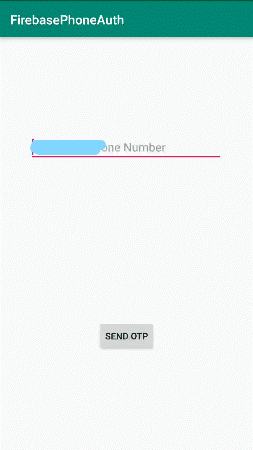
Introduction
In this blog I'm going to show you how to use Phone Authentication using Firebase in Android.
What is Firebase ?
Firebase is a Google's mobile platform which provides backend services like database , storage , authentication etc. Firebase supports authentication using email , phone number , Google , Twitter and many more. In this blog we will be covering the Phone Authentication only.
Sample App
We will build a simple app which contains three activities :
- MainActivity: In this activity user will enter his/her phone Number
- OtpActivity: In this activity user will enter the otp received on his/her phone for verification.
- HomeActivity: After user is successfully logged in then this activity will be displayed.
Enough of theory , Let's begin the code.
Setup Firebase
To setup Firebase in your Android project , you need to follow these steps :
- Open FirebaseConsole and login to your account.
- On welcome screen , Click on "Add project" option.
- After clicking on "Add Project" option following project window will open , in this enter your project name and your country and then click on "CREATE PROJECT" button.
-
After this your project's dashboard will open from here click on "Add Firebase to your Android project".
- After clicking on "Android icon" you need to register your app by providing the app package name and SHA-1 signing certificate.
- To get the app's package name , open AndroidManifest.xml file and on the top there is a package name mentioned.
- To get the SHA-1 certificate , click on "Gradle" tab on right side on Android studio then click on "app" -> "android" -> double click on "signing report". In monitor pane you will get your SHA-1 certificate.
- After clicking on "Register App" button you have to download "google-services.json" file and paste it in app module.
-
Now , open Project level build.gradle file and add the following code under dependencies section.
- Open app level build.gradle file and add the following code under dependencies section.
- Now , go back to Firebase console and click on "Project Overview" . In "Discover Firebase" section click on "Authentication" then on next page click on "Setup Sign in method and enable phone authentication and save it."
That's all we are done with the initial setup.
Adding Permissions
Open AndroidManifest.xml file and add the following code , We need Internet permission for sending otp.
Designing Layout
Open activity_main.xml file and add the following code , This contains one editText for entering phone number , button for sending otp and one progress bar.
Setting Up MainActivity
Open MainActivity.java file and add the following code . We are creating objects of our UI elements and finding the reference to all of the objects using findViewById() method.
Send Verification Code
Open MainActivity.java file and add the following code . On Click of button first retreive the text from editText and pass this string to our custom method called "sendVerificationCode()".
What is PhoneAuthProvider ?
PhoneAuthProvider is a class which represents the whole mechanism for phone authentication and it also provides some methods and callback functions such as "verifyPhoneNumber()".
What is verifyPhoneNumber() ?
verifyPhoneNumber is a function which starts the phone number verification process , this takes following arguments :
- phoneNumber: User's phone number for which verification has to be start.
- timeout: Timeout duration of verification code or otp.
- unit: Unit of timeout , can be in seconds , milliseconds etc.
- activity or context: Context from where the method is called.
- OnVerificationStateChangedCallbacks: Instanc of OnVerificationStateChangedCallbacks which contains the callback functions that handle the result of request.
OnVerificationStateChangedCallbacks
This is an abstract class which contains some callback functions for different phone auth events . It is mandatory to provide implementation of two callback functions . Following callback functions can be used :
- onVerificationCompleted: Called when verification is donw without user interaction , ex- when user is verified withot code.
- onVerificationFailed: Called when some error occured such as failing of sending SMS or Number format exception.
- onCodeSent: Called when verification code is successfully sent to the phone number.
On Click of button we are calling our "sendVerificationCode" function which will be responsible for sending sms to user's phone number , when onCodeSent() is triggered the control will be transferred to OtpActivity where we can verify the SMS.
Setting Up OtpActivity
Now , create new empty activity named OtpActivity and add the following code inside this file and for the layout add the xml code in activity_otp.xml file.
OtpActivity layout contains one editText for otp , one button for verifying the otp and one progress bar.
In OtpActivity.java file we are receiving intent and verificationId from MainActivity , verificationId is required for PhoneAuthCredential object.
Verify Otp
Open OtpActivity.java file and add the following code , on click of a button we are calling our custom method named "verifyOtp".
What is PhoneAuthCredential ?
PhoneAuthCredential is a class which wraps the phone number and other verification information such as verificationId to create a credential and then this credential is passed to another method named "signInWithCredential".
What is signInWithCredential ?
signInWithCredential is a function which is used to sign in the user based on the credential it receives , then we can attach the "onCompleteListener" to this method so that based on the result whether it's success of failure we can perform our operations in this case sending an intent to HomeActivity when user is successfully signed in and displaying the toast in case of any errors.
Setting Up HomeActivity
Now , create new empty activity named HomeActivity and add the following code inside this file and for the layout add the xml code in activity_home.xml file . Layout contains only onw Welcome text.
That's All! Happy Coding!
The complete code is available here.
Staying in the Loop
If you want to stay in the loop and get an email when I write new blog posts, Follow me on Instagram or join the CodingWithMitch community on my website. It only takes about 30 seconds to register.
Authors
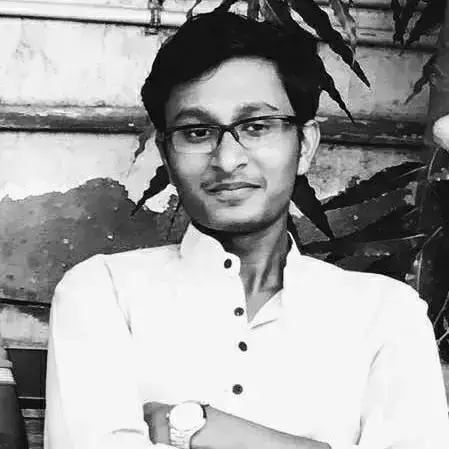
Create an Account
Similar Posts
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
Comments