Introduction
In this post I show you how to use a Staggered RecyclerView to display list items with an image and text.
You'll learn to build a recyclerview adapter class from scratch and display images and text. To retrieve the images from the internet we use the "Glide" library.
Make sure to get the most up-to-date version of the Glide library. Outdated versions typically have issues. You can get the dependency here: https://github.com/bumptech/glide
This is a perfect example if you're a beginner and need a step-by-step example on how to use a staggered recyclerview in your android project.
All Source Code is available on my Github for free.
App Demo
Here's a visual of the simple app we'll be building:
The app will have a single activity: MainActivity.java
MainActivity will contain a RecyclerView with all the list items.
Dependencies
We'll start with the dependencies:
We have:
- The RecyclerView support library that's required for using RecyclerViews
- The CardView support library for using CardViews
- The Glide library for retrieving and displaying images from the internet
- And a CircleImageView library for displaying circular images
Remember to get the most up-to-date version of the Glide library: https://github.com/bumptech/glide
build.gradle (app file)
Layouts
Next we have the layouts.
activity_main.xml
activity_main is simple. There's just a RecyclerView widget with a vertical orientation for holding our list items.
layout_grid_item.xml
layout_grid_item is responsible for displaying the individual list items in the RecyclerView. It contains a CardView, an ImageView, and a TextView widget.
Java Classes
We have two java classes:
- StaggeredRecyclerViewAdapter.java
- MainActivity.java
StaggeredRecyclerViewAdapter.java
The StaggeredRecyclerViewAdapter class is for adapting our individual list items to the RecyclerView list.
Notice there's nothing different here as compared to a regular RecyclerView adapter class. There's nothing special you need to do in the adapter to make it "staggered". All the difference will be in MainActivity.
MainActivity.java
Notice the creation of a "StaggeredGridLayoutManager" object in the initRecyclerView method.
That's essentially the only difference between a regular RecyclerView and a Staggered RecyclerView.
Internet Permissions
Don't forget to add internet permission to the manifest.
Summary
Integrating Staggered RecyclerViews is almost the same exact process as integrating a regular RecyclerView. As you've seen, the only real difference is the presence of a "StaggeredGridLayoutManager" object.
Staying in the Loop
If you want to stay in the loop and get an email when I write new blog posts, Follow me on Instagram or join the CodingWithMitch community on my website. It only takes about 30 seconds to register.
Authors
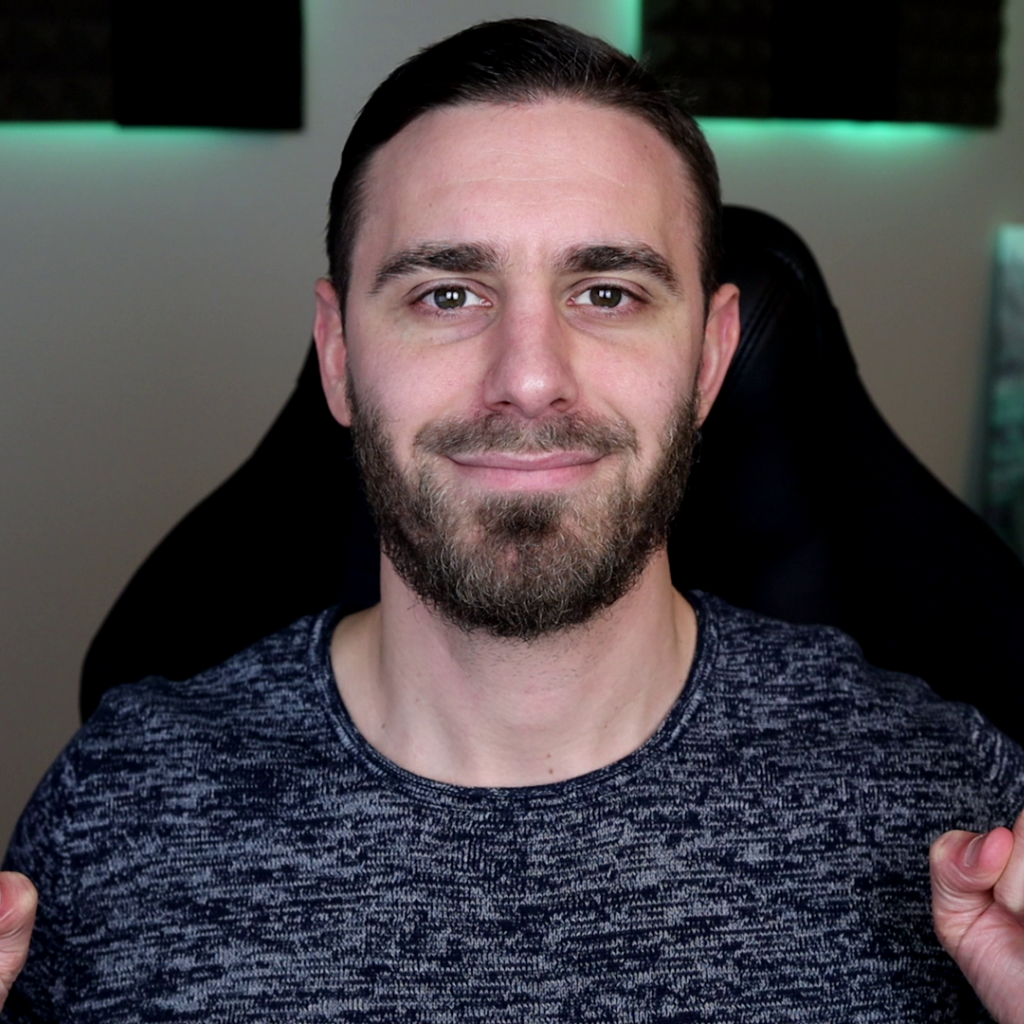
Create an Account
Similar Posts
Playing Video in a RecyclerView with ExoPlayer
March 4, 2019
RecyclerView
March 4, 2019
Filtering a RecyclerView with SearchView
March 4, 2019
Kotlin RecyclerView Template (DiffUtil and AsyncListDiffer)
Sept. 13, 2019
RecyclerView OnClickListener
March 4, 2019
Horizontal RecyclerView
March 4, 2019
SQLite and the Room Persistence Library
March 4, 2019
Swiping Views with ViewPager
March 4, 2019
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
Comments