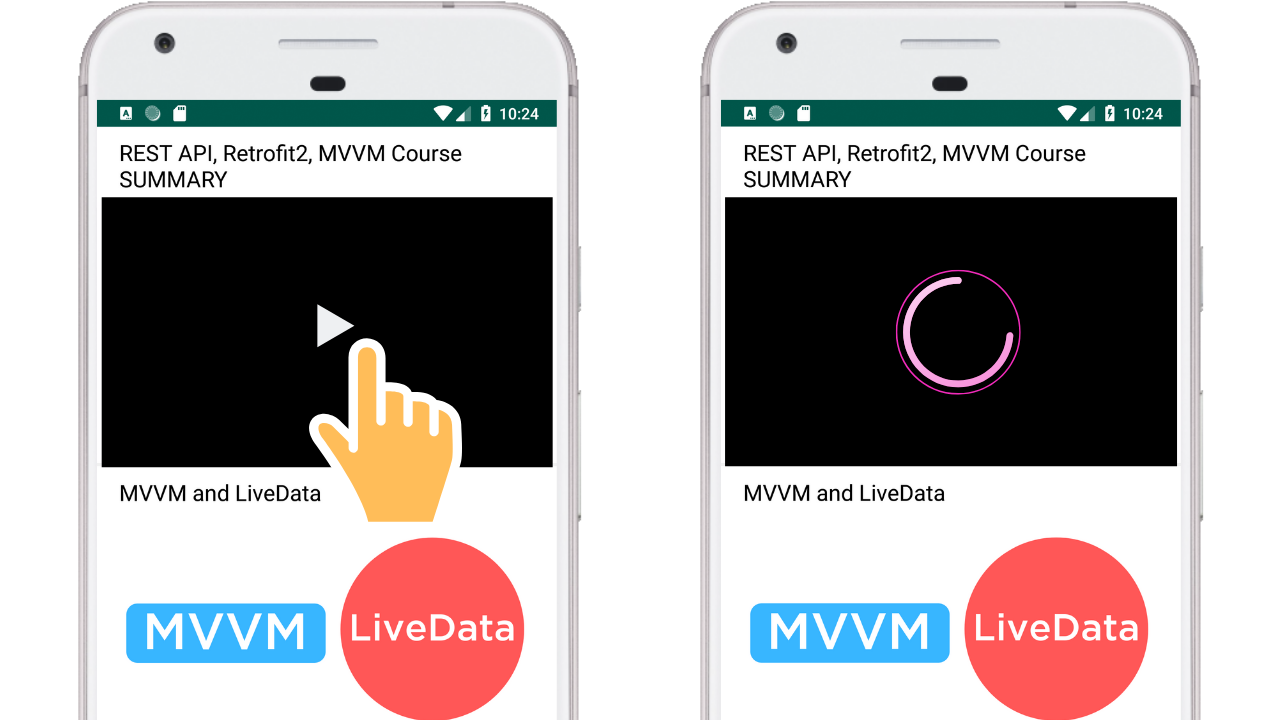
Table of Contents:
- Demo
- Introduction
- Dependencies
- Modeling the Data
- Resources
- Drawables
- RecyclerView List-item Layout
- ViewHolder
- RecyclerView Adapter
- Custom RecyclerView
- activity_main.xml
- RecyclerView Item Decoration
- MainActivity
- Final Thoughts and Complete Source Code
If you prefer to watch video as opposed to read, I made a YouTube tutorial: watch the tutorial.
Demo
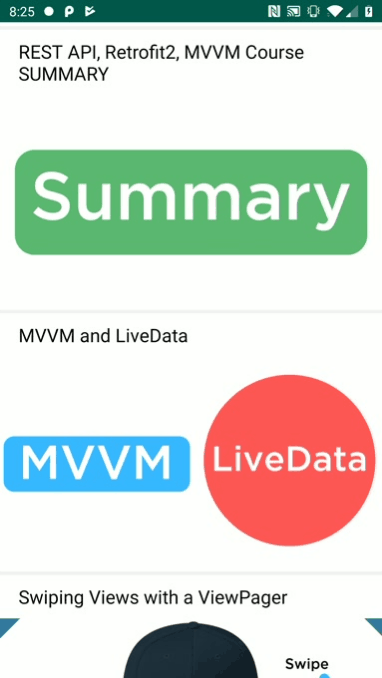
Introduction
Playing video in a RecyclerView seems like something that should be simple. So many popular apps do it: Instagram, YouTube, Twitter, the list goes on.
It turns out, it's not that simple. The Android SDK doesn't have an "out of the box" solution. So you'll need to build something custom.
In this blog post I'll guide you through how to build a custom RecyclerView, ViewHolder, and RecyclerView Adapter that will allow you to implement the functionality shown in the demo above.
Dependencies
I need three external dependencies to build this app:
- The RecyclerView dependency
- Glide for displaying images
- And ExoPlayer for playing video
Modeling the Data
When building any sort of custom functionality, one of the first steps is usually figuring out what kind of data you'll be using. In the context of this post, that means figuring out what kind of objects I'll be displaying as list-items in the RecyclerView.
I think these fields are self-explanatory. But just to clarify, the
media_url
field is for the video url. That's what I'll be passing to ExoPlayer to play the video.
Resources
Now that I have a data model, I can add some static resources. These will be the list-items. Feel free to add different resources for your project.
Drawables
You'll need to add three drawables to your projects drawables folder. Here's a link where you can get them. Get the drawables
RecyclerView List-item Layout
There will be two layout files:
-
activity_main.xml
This will contain the custom RecyclerView. -
layout_video_list_item.xml
This is the layout for the RecyclerView list-items.
Just make the list-item layout for now. You can't add the custom RecyclerView to activity_main.xml yet because we haven't built it.
ViewHolder
Now that I have a list-item layout for the RecyclerView, I can build the ViewHolder class.
RecyclerView Adapter
The only thing I'm passing into the adapter is a reference to the Glide instance, and a list of MediaObjects.
This RecyclerView adapter is probably much less complicated than you were expecting. I'm guessing you thought all the logic for playing video was going to be in this class. I definitely could have done that. But putting all the video code in a custom RecyclerView class is much cleaner.
That's what I'll work on next.
Custom RecyclerView
This is the most difficult part of the post. The custom RecyclerView class is where all the logic lives for preparing the ExoPlayer and deciding what video to play.
If you want to hear me explain this class in detail, I made a video tutorial of this post on YouTube. Watch the tutorial.
activity_main.xml
Now that the custom RecyclerView has been built, I can add it to activity_main.xml.
RecyclerView Item Decoration
I added some very minor styling to the RecyclerView. Just some spacing between the list-items. To add the spacing I created a custom ItemDecoration class. In the section below (MainActivity), I'll setup the RecyclerView and add the ItemDecoration.
MainActivity
This is the last step. I need to:
- Release the ExoPlayer if the activity is destroyed
- Initialize the Glide instance with some default RequestOptions.
- And initialize the RecyclerView.
Now run it and test. You should see an output like what's shown in the demo above.
If you're having trouble, watch the YouTube tutorial where I code this on video.
Final Thoughts and Complete Source Code
I think it's strange that the Android SDK doesn't have some kind of default implementation that facilitates video playback in a RecyclerView. Even something that makes it easier to figure out what list-item is currently being viewed by the user would be nice.
Hopefully this helped you add something cool to your projects.
The complete code is available here.
Staying in the Loop
If you want to stay in the loop and get an email when I write new blog posts, Follow me on Instagram or join the CodingWithMitch community on my website. It only takes about 30 seconds to register.
Authors
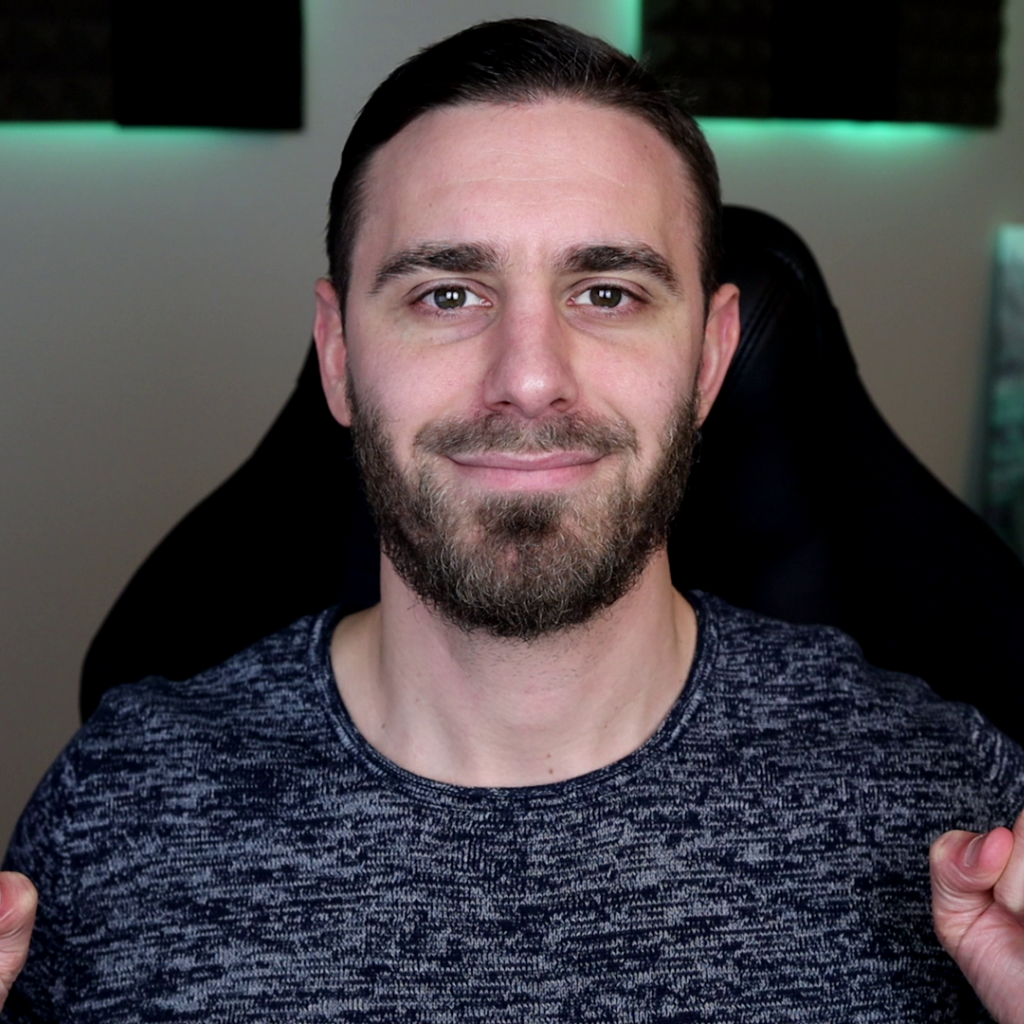
Create an Account
Similar Posts
RecyclerView
March 4, 2019
Getting Started with ExoPlayer on Android
March 4, 2019
Filtering a RecyclerView with SearchView
March 4, 2019
Kotlin RecyclerView Template (DiffUtil and AsyncListDiffer)
Sept. 13, 2019
RecyclerView OnClickListener
March 4, 2019
Staggered RecyclerView
March 4, 2019
Horizontal RecyclerView
March 4, 2019
Swiping Views with ViewPager
March 4, 2019
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
Comments