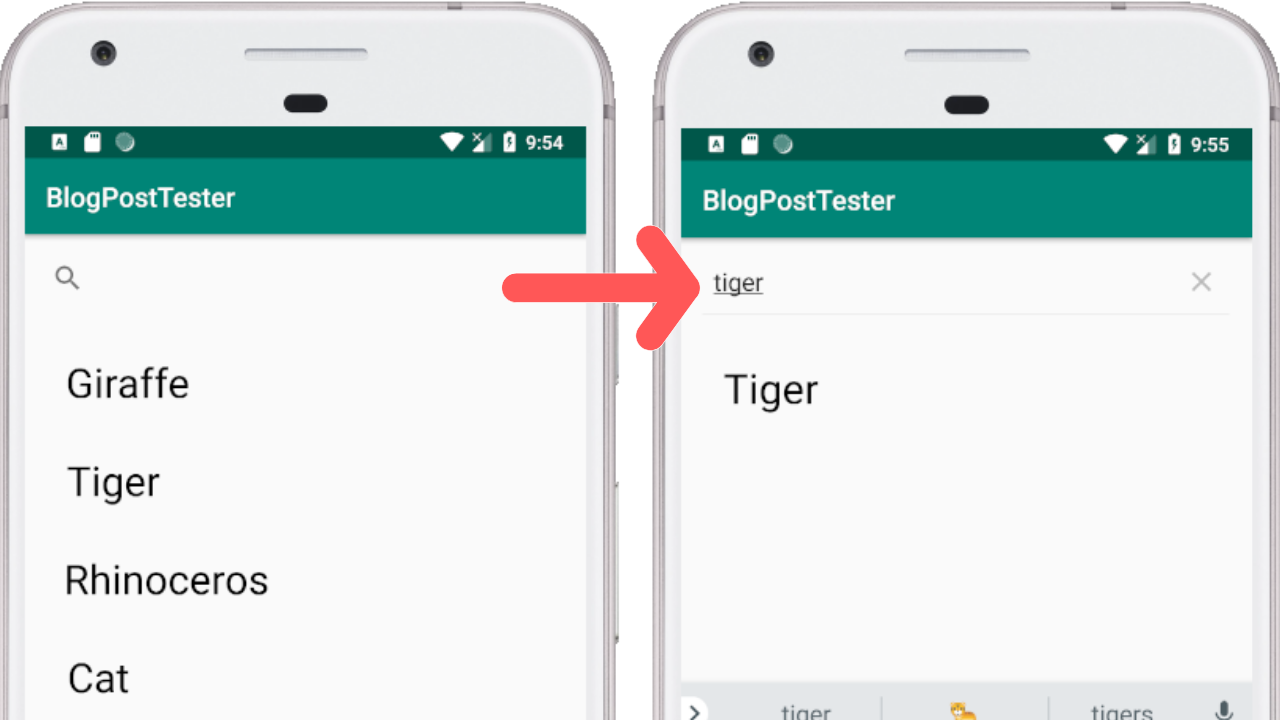
Table of Contents:
- Demo
- Introduction
- What is a SearchView?
-
An Example:
Demo
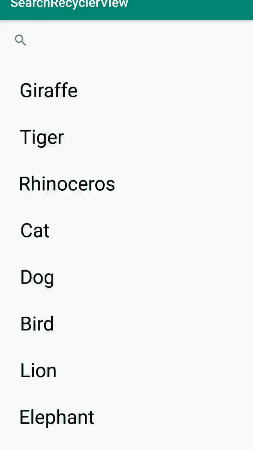
Introduction
In this blog I'm going to show you how to filter a RecyclerView using a SearchView on Android
What is a SearchView?
SearchView : A SearchView is a widget used to capture text input. Usually it gets placed inside a Toolbar. That text input can then be used for a number of different tasks. In this blog post I'm going to show you how to use a SearchView to filter results in a RecyclerView.
For more information on RecyclerView's, check out this blog post: RecyclerView
Dependencies
Open build.gradle (Module:app) and add RecyclerView dependency.
Make sure you have the latest version of dependencies. You can check the latest version here
Designing MainActivity Layout
Add the following code in your activity_main.xml file. This layout contains SearchView and a RecyclerView. SearchView is a widget that provides a user interface for the user to enter a search query and submit a request to a search provider
Model
Make a model class Name.java and put the following code inside it. This model class denotes name as the data member which contains the animal name
Designing List Layout
To display the Animals Name on the screen, we need to design the layout of the list item. This layout file contains the one TextView which will display the name of the Animal
Implementing Search in Adapter class
The First step is to implements the Filterable interface and override the getFilter() method and Declare the two List of type "Name" class. The first list is an initial list which contains all the elements and the second list (as the name suggests) will contain the items which are filtered according to the query typed in the Searchview and pass it in the constructor.
The second step is to write search operation logic in getFilter() method. Inside getFilter() method, return an instance of Filter which will then Override two methods named performFiltering() and publishResults().
- performFiltering() :
This Method is invoke in a worker thread and it is used to filter the results according to the constraint specified. The main logic inside this method is that we are going through each object of Name class inside nameList and comparing the name of the animal with the query typed, If both becomes equal then we are adding the updated elements inside the new List named "filteredList" and assigning the "filteredList" to "filteredNameList" and at last we are returning the filtered results to the publishResults() method.
- publishResults() :
This method is invoked in the UI thread and it is used to publish the filtering results in the user interface. Inside this method, we are assigning the value of filtered list to the "filteredNameList" and notifying the observers using notifyDataSetChanged() method.
Complete Recyclerview Adapter Class
This is a complete Adapter class which contains the search logic which is explained above.
Adding the Recyclerview in Activity
Now, Declare all these objects inside MainActivity before onCreate() method and then, we will add some Animal Names to the list and set up the Adapter with RecyclerView inside onCreate() method.
onQueryTextChangeListener
Finallly, we will setup the SearchView inside the MainActivity. Paste the below code inside the onCreate() method of MainActivity. We are attaching the listener on SearchView and Overrriding two methods.
- onQueryTextSubmit(String queryString) :
This method is invoked when then the search button is pressed to submit the query. In this method, we are passing the queryString to the filter() method which will start filtering the results according to the query passed.
- onQueryTextChange(String queryString) :
This method is invoked when something is changed in the Searchview. In this method also, we are passing the queryString to the filter() method which will start filtering the results according to the query passed.
That's All! Happy Coding!
The complete code is available here.
Staying in the Loop
If you want to stay in the loop and get an email when I write new blog posts, Follow me on Instagram or join the CodingWithMitch community on my website. It only takes about 30 seconds to register.
Authors
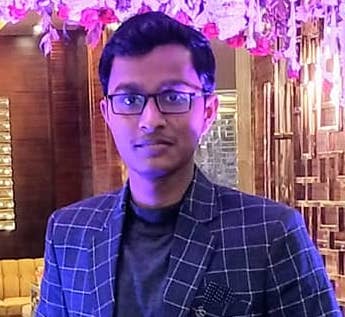
Create an Account
Similar Posts
Playing Video in a RecyclerView with ExoPlayer
March 4, 2019
RecyclerView
March 4, 2019
Kotlin RecyclerView Template (DiffUtil and AsyncListDiffer)
Sept. 13, 2019
RecyclerView OnClickListener
March 4, 2019
Staggered RecyclerView
March 4, 2019
Horizontal RecyclerView
March 4, 2019
Swiping Views with ViewPager
March 4, 2019
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
CodingWithMitch Members
Unlimited access to all courses and videos
Step by step guides to build real projects
Video downloads for offline viewing
Members can vote on what kind of content they want to see
Access to a private chat with other communnity members & Mitch
Comments