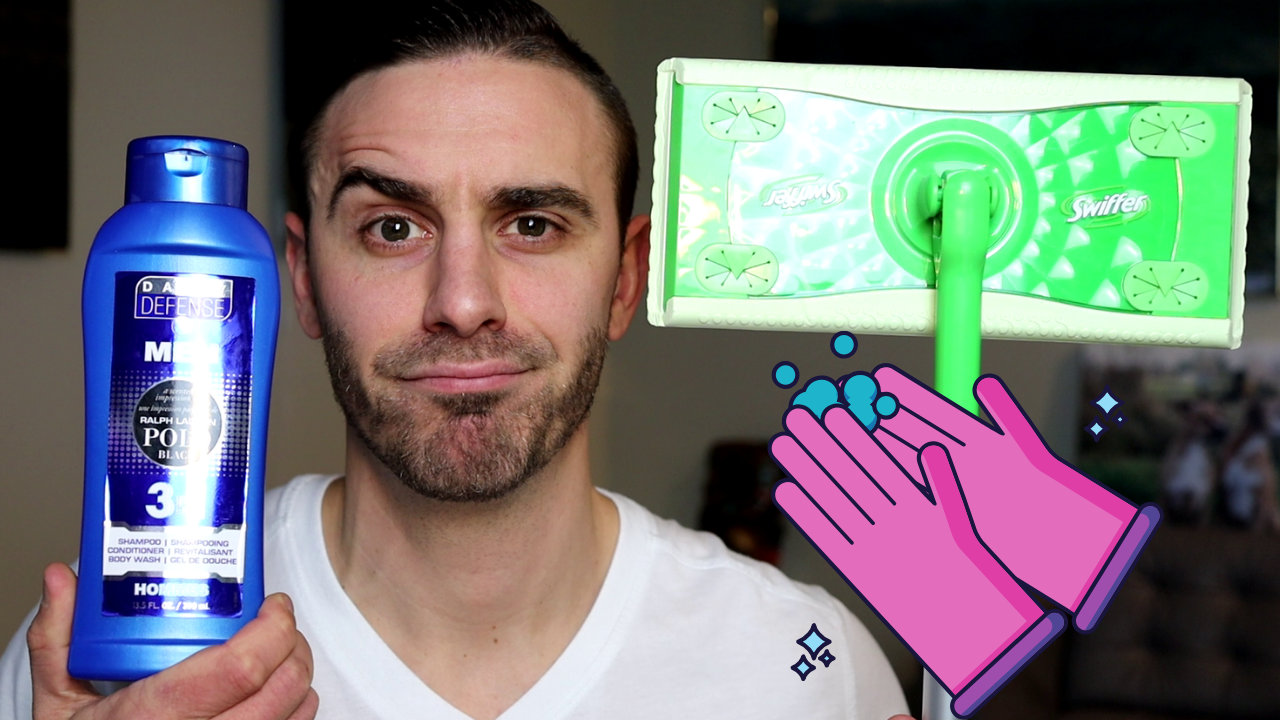
Clean Architecture
Watch demoDescription
Course Objective:
Why do so many people from the various software fields (web, android, iOS, desktop, games) say Clean Architecture is one of the best ways to structure code? This course will introduce you to Clean Architecture and show you how it makes testing far easier on android. Both Instrumentation tests and unit tests.
- MVI architectural pattern
- Kotlin
- Coroutines
- Flows and channels
- Network layer (Firestore)
- Caching layer (Room Persistence) & planning a caching strategy
- Firestore testing (Firebase Emulators)
- Unit tests (Junit5)
- Instrumentation testing (Espresso and androidx.test)
- Gradle Scripting (automate test running)
- Test reports
- Dagger2
- Navigation Components
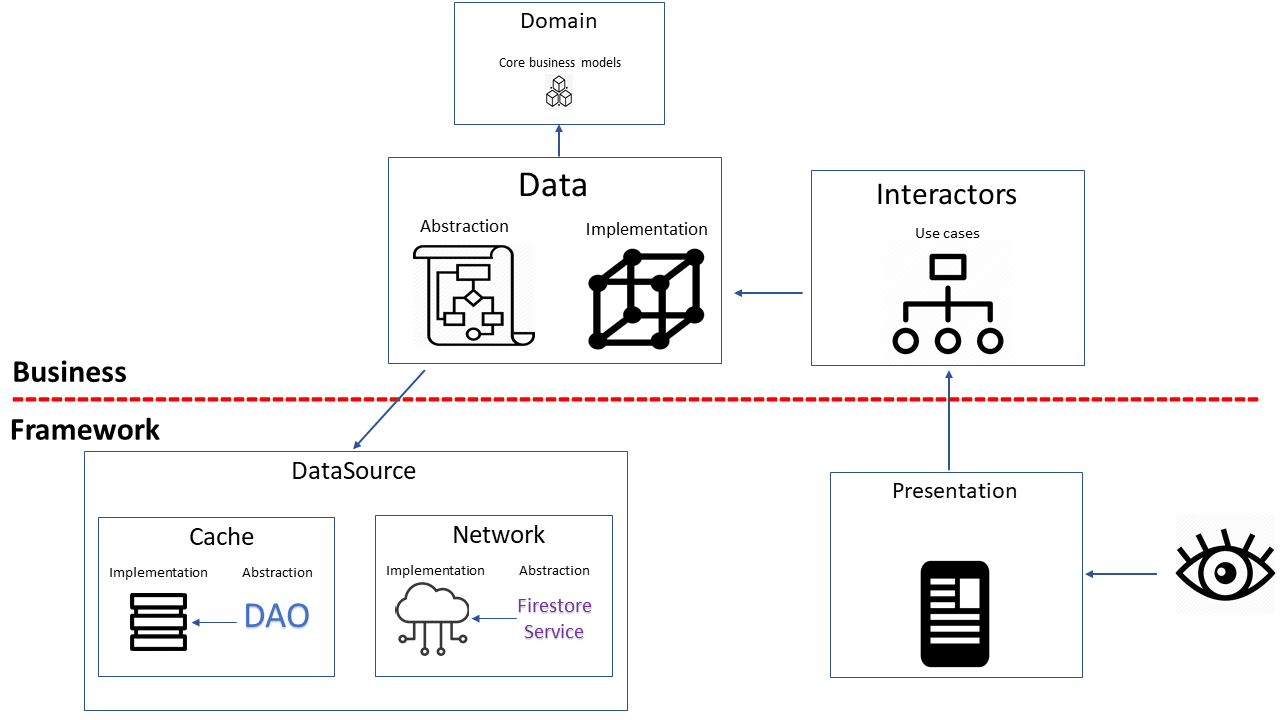
Target Audience:
This is not a beginner course. See prerequisites section below.
Prerequisites:
Note: These are recommendations, not requirements. I think you can get by since I explain everything on video anyway.
- Kotlin
- Dagger2
- Room Persistence
- Retrofit2
- MVI / MVVM Architecture
- Unit testing
- Instrumentation testing
Metadata
Published: Aug. 2, 2021
Lectures: 70
Total video time: 10:53:36
Authors
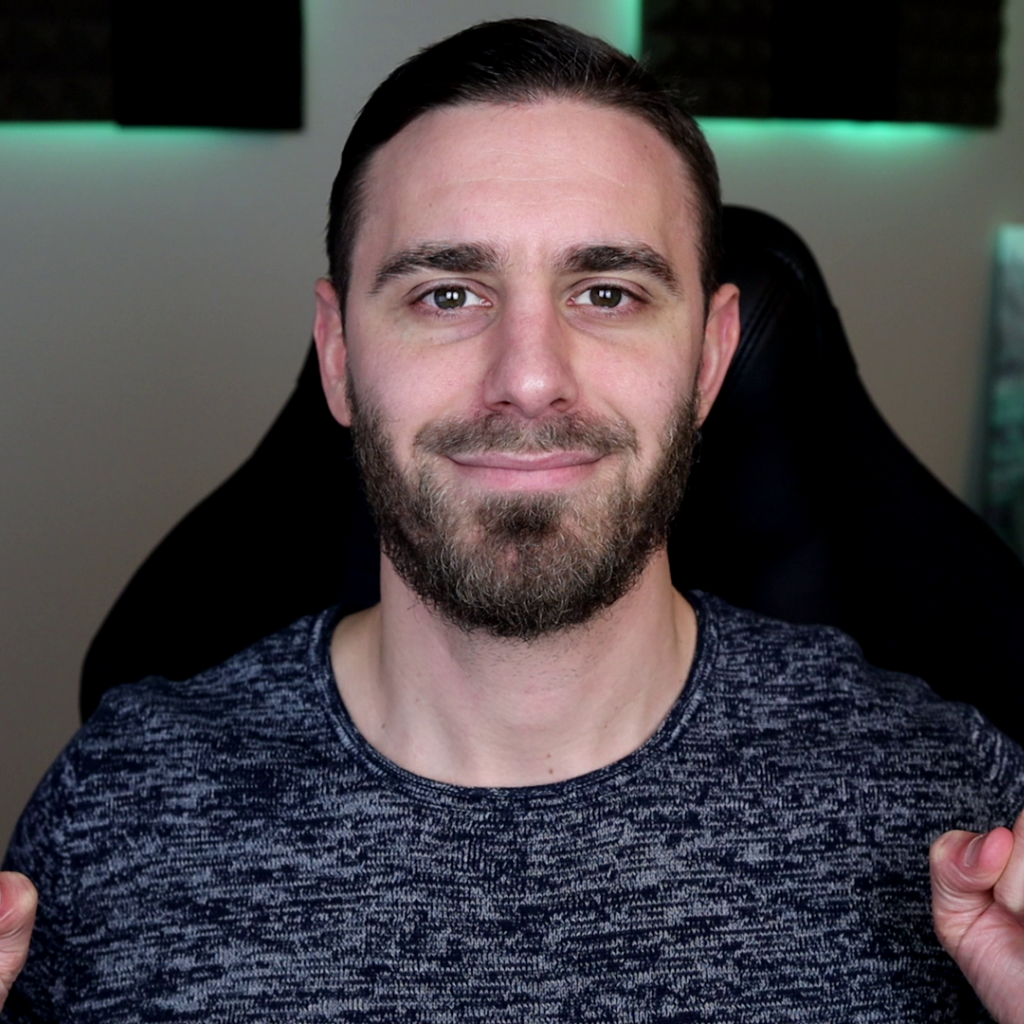
Clean Architecture
If you restart this course, all your progress will be reset
Lectures
-
Course Demo
-
Part 1: How this Course is Structured
-
How this Course is StructuredFREE
-
Source CodeFREE
-
Part 2: Gradle Dependency Management
-
Gradle Dependency ManagementFREE
-
Part 3: Layers of Clean Architecture
-
Layers of Clean ArchitectureFREE
-
Package StructureFREE
-
Part 4: Domain Layer
-
Building out the Domain Layer
-
State Management
-
Part 5: Data Layer
-
Data Layer (Cache)
-
Data Layer (Network)
-
Part 6: Use-cases
-
NoteDaoService & NoteFirestoreService Abstractions
-
Planning Use-cases
-
InsertNewNote Use-case
-
Cache and Network Error Handling
-
Test Fakes and Test Dependencies
-
InsertNewNote Unit Tests
-
SearchNotes Use-case
-
SearchNotes Unit Tests
-
Fake Data Sets
-
GetNumNotes Use-case
-
GetNumNotes Unit Tests
-
DeleteNote Use-case
-
DeleteNote Unit tests
-
DeleteMultipleNotes Use-case
-
DeleteMultipleNotes Unit Tests
-
RestoreDeletedNote Use case
-
RestoreDeletedNote Unit Tests
-
UpdateNote Use-case
-
UpdateNote Unit Tests
-
SyncNotes Use-case
-
SyncNotes Unit Tests
-
SyncDeletedNotes Use-case
-
SyncDeletesNotes Unit Tests
-
Part 7: DataSource Caching Layer
-
Room Database Setup
-
NoteDaoService Implementation
-
Part 8: DataSource Network Layer
-
Firestore Setup
-
Datasource Network Layer Setup
-
Crashlytics and Analytics
-
Part 9: Dagger Setup
-
Dagger Setup (Part 1)
-
Dagger for Instrumentation Tests
-
Part 10: Testing a Firestore Database
-
Firestore Local Testing
-
Base Test Class
-
Fake Data for Instrumentation Tests
-
Firestore Tests
-
Bug Fix #1
-
Part 11: Testing a Room Database
-
Room DAO Tests
-
Part 12: Gradle Scripts and Test Reports
-
Gradle Scripting Introduction
-
Gradle Scripts to Automate Tests
-
Part 13: Interactors, ViewModels and UI
-
The Last Piece of Clean Architecture
-
Base Classes and Factories
-
NoteListViewModel
-
NoteList Toolbar States and RecyclerView Item Selection
-
NoteListFragment
-
MainActivity
-
NoteDetailViewModel and Fragment
-
SplashFragment and Data Sync
-
Part 14: Bug Fixes and Refactoring
-
Bug Fixes 2
Comments
Lectures
-
Course Demo
-
Part 1: How this Course is Structured
-
How this Course is StructuredFREE
-
Source CodeFREE
-
Part 2: Gradle Dependency Management
-
Gradle Dependency ManagementFREE
-
Part 3: Layers of Clean Architecture
-
Layers of Clean ArchitectureFREE
-
Package StructureFREE
-
Part 4: Domain Layer
-
Building out the Domain Layer
-
State Management
-
Part 5: Data Layer
-
Data Layer (Cache)
-
Data Layer (Network)
-
Part 6: Use-cases
-
NoteDaoService & NoteFirestoreService Abstractions
-
Planning Use-cases
-
InsertNewNote Use-case
-
Cache and Network Error Handling
-
Test Fakes and Test Dependencies
-
InsertNewNote Unit Tests
-
SearchNotes Use-case
-
SearchNotes Unit Tests
-
Fake Data Sets
-
GetNumNotes Use-case
-
GetNumNotes Unit Tests
-
DeleteNote Use-case
-
DeleteNote Unit tests
-
DeleteMultipleNotes Use-case
-
DeleteMultipleNotes Unit Tests
-
RestoreDeletedNote Use case
-
RestoreDeletedNote Unit Tests
-
UpdateNote Use-case
-
UpdateNote Unit Tests
-
SyncNotes Use-case
-
SyncNotes Unit Tests
-
SyncDeletedNotes Use-case
-
SyncDeletesNotes Unit Tests
-
Part 7: DataSource Caching Layer
-
Room Database Setup
-
NoteDaoService Implementation
-
Part 8: DataSource Network Layer
-
Firestore Setup
-
Datasource Network Layer Setup
-
Crashlytics and Analytics
-
Part 9: Dagger Setup
-
Dagger Setup (Part 1)
-
Dagger for Instrumentation Tests
-
Part 10: Testing a Firestore Database
-
Firestore Local Testing
-
Base Test Class
-
Fake Data for Instrumentation Tests
-
Firestore Tests
-
Bug Fix #1
-
Part 11: Testing a Room Database
-
Room DAO Tests
-
Part 12: Gradle Scripts and Test Reports
-
Gradle Scripting Introduction
-
Gradle Scripts to Automate Tests
-
Part 13: Interactors, ViewModels and UI
-
The Last Piece of Clean Architecture
-
Base Classes and Factories
-
NoteListViewModel
-
NoteList Toolbar States and RecyclerView Item Selection
-
NoteListFragment
-
MainActivity
-
NoteDetailViewModel and Fragment
-
SplashFragment and Data Sync
-
Part 14: Bug Fixes and Refactoring
-
Bug Fixes 2