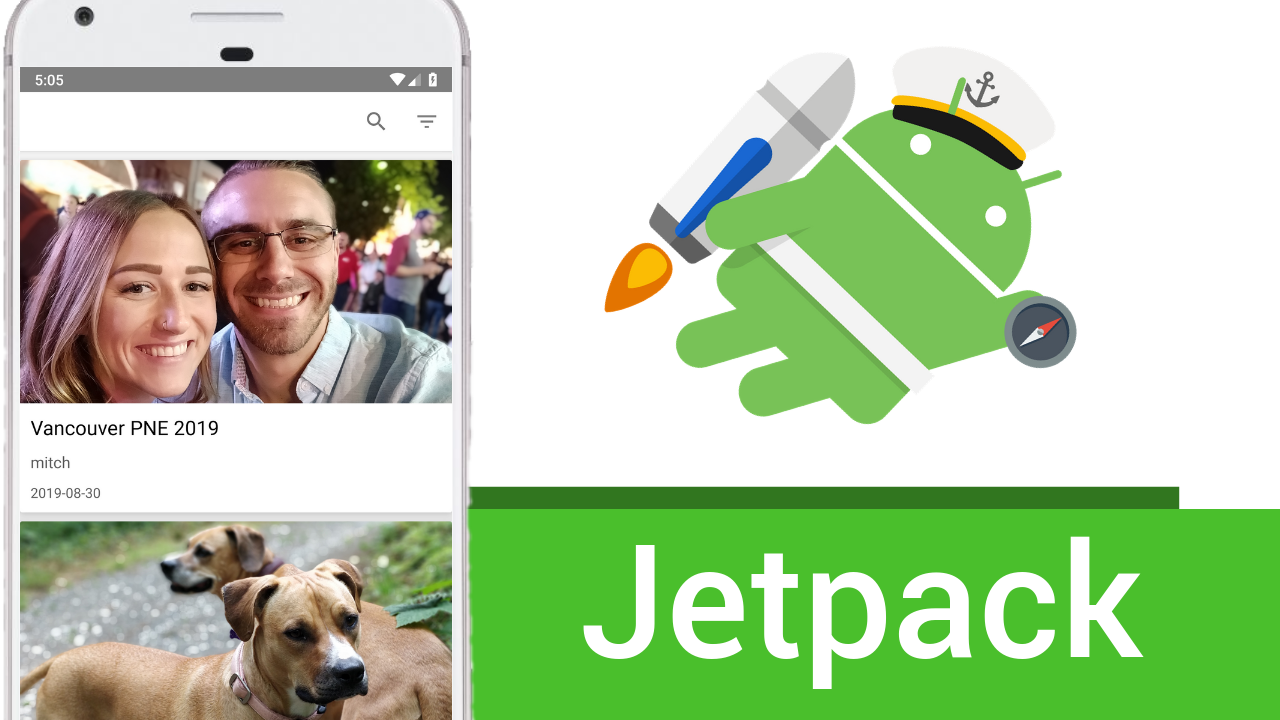
Powerful Android Apps with Jetpack Architecture
Watch demoDescription
In this course you'll learn to build a real application that interacts with the website open-api.xyz.
Open-api.xyz is a sandbox website for codingwithmitch members to practice interacting with a Rest API.
Prerequisites (Recommended not required):
What you'll learn:
- Kotlin:
-
Coroutines:
- Advanced coroutine management using jobs
- Cancelling active jobs
- Coroutine scoping
-
Navigation Components:
- Bottom Navigation View with fragments
- Leveraging multiple navigation graphs (this is cutting edge content)
-
Dagger 2:
- custom scopes, fragment injection, activity injection, Viewmodel injection
-
MVI architecture:
- Basically this is MVVM with some additions
- State management
- Building a generic BaseViewModel
- Repository pattern (NetworkBoundResource)
-
Room Persistence:
- SQLite on Android with Room Persistence library
- Custom queries, inserts, deletes, updates
- Foreign Key relationships
- Multiple database tables
-
Cache:
- Database caching (saving data from network into local cache)
- Single source of truth principal
-
Retrofit 2:
- Handling any type of response from server (success, error, none, etc...)
- Returning LiveData from Retrofit calls (Retrofit Call Adapter)
-
ViewModels:
- Sharing a ViewModel between several fragments
- Building a powerful generic BaseViewModel
-
WebViews:
- Interacting with the server through a webview (Javascript)
-
SearchView:
- Programmatically implement a SearchView
- Execute search queries to network and db cache
-
Images:
- Selecting images from phone memory
- Cropping images to a specific aspect ratio
- Setting limitations on image size and aspect ratio
- Uploading a cropped image to server
-
Network Request Management:
- Cancelling pending network requests (Kotlin coroutines)
- Testing for network delays
-
Pagination:
- Paginating objects returned from server and database cache
-
Material Design:
- Bottom Navigation View with Fragments
- Customizing Bottom Navigation Icon behavior
- Handling Different Screen Sizes (ConstraintLayout)
- Material Dialogs
- Fragment transition animations
Github repo: Open-Api Android App
Metadata
Published: Aug. 2, 2021
Lectures: 61
Total video time: 18:22:64
Authors
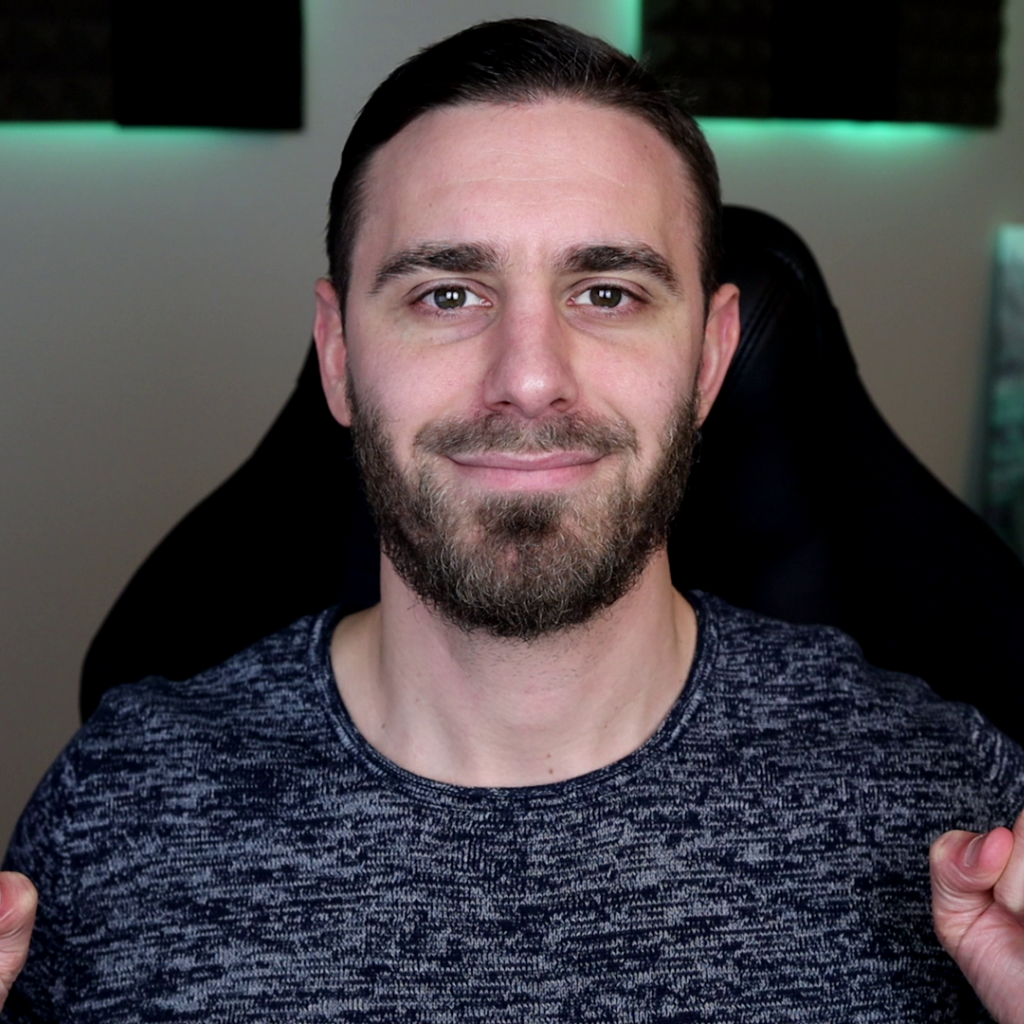
Powerful Android Apps with Jetpack Architecture
If you restart this course, all your progress will be reset
Lectures
-
Course Demo
-
Starting Point and DependenciesFREE
-
Begin Part 1
-
Part 1 IntroductionFREE
-
Navigation Graphs for AuthenticationFREE
-
Architectural SetupFREE
-
Room Persistence SetupFREE
-
Dagger SetupFREE
-
Retrofit SetupFREE
-
MVI Architecture Generics (DataState, Events, Wrappers)FREE
-
AuthViewModel, AuthViewState and AuthStateEvent
-
Session Manager and Authentication
-
Login and Registration
-
NetworkBoundResource for MVI and Coroutines
-
Leveraging NetworkBoundResource
-
Handling Errors and Loading
-
Auto-Authentication when App Launches
-
Password Reset
-
Begin Part 2
-
Part 2 Introduction
-
Main Package Fragments and Layouts
-
Bottom Navigation Graphs
-
Custom Bottom Nav Controller
-
Navigation Controller and Toolbar
-
Begin Part 3
-
Part 3 Introduction
-
Account: ViewModel, State, Repository and ApiService
-
Caching Account Properties
-
Updating Account Properties
-
Password Update
-
Keyboard Management
-
Job Manager and Cancelling Jobs
-
Begin Part 4
-
Part 4 Introduction
-
Blog: ViewModel, State, Repository and ApiService
-
Retrieving and Caching Blog Posts from Network
-
RecyclerView Adapter, DiffUtil and ListUpdateCallback
-
Selecting a Blog Post
-
Pagination
-
SearchView and Swipe Refresh
-
Filtering and Ordering (custom Room queries)
-
Material Dialogs Custom Layout
-
Determining if Author of BlogPost
-
Deleting Blog Posts
-
Are You Sure? (Dialog)
-
Updating Blog Posts
-
On Blog Update Success
-
Image Cacheing with Glide (Preloader)
-
Begin Part 5
-
Part 5 Introduction
-
CreateBlog: ViewModel, State and Repository
-
Selecting and Cropping an Image
-
Uploading Image to Server with Retrofit
-
Updating a Blog Post
-
Part 6: Refactoring
-
Dagger Refactor
-
Process Death Issue Fix (Part 1/2)
-
Process Death Issue Fix (Part 2/2)
-
BackStack Bug
-
Navigation Bug
-
Remove dagger-android
-
Flows and Channels Refactor
Comments
Lectures
-
Course Demo
-
Starting Point and DependenciesFREE
-
Begin Part 1
-
Part 1 IntroductionFREE
-
Navigation Graphs for AuthenticationFREE
-
Architectural SetupFREE
-
Room Persistence SetupFREE
-
Dagger SetupFREE
-
Retrofit SetupFREE
-
MVI Architecture Generics (DataState, Events, Wrappers)FREE
-
AuthViewModel, AuthViewState and AuthStateEvent
-
Session Manager and Authentication
-
Login and Registration
-
NetworkBoundResource for MVI and Coroutines
-
Leveraging NetworkBoundResource
-
Handling Errors and Loading
-
Auto-Authentication when App Launches
-
Password Reset
-
Begin Part 2
-
Part 2 Introduction
-
Main Package Fragments and Layouts
-
Bottom Navigation Graphs
-
Custom Bottom Nav Controller
-
Navigation Controller and Toolbar
-
Begin Part 3
-
Part 3 Introduction
-
Account: ViewModel, State, Repository and ApiService
-
Caching Account Properties
-
Updating Account Properties
-
Password Update
-
Keyboard Management
-
Job Manager and Cancelling Jobs
-
Begin Part 4
-
Part 4 Introduction
-
Blog: ViewModel, State, Repository and ApiService
-
Retrieving and Caching Blog Posts from Network
-
RecyclerView Adapter, DiffUtil and ListUpdateCallback
-
Selecting a Blog Post
-
Pagination
-
SearchView and Swipe Refresh
-
Filtering and Ordering (custom Room queries)
-
Material Dialogs Custom Layout
-
Determining if Author of BlogPost
-
Deleting Blog Posts
-
Are You Sure? (Dialog)
-
Updating Blog Posts
-
On Blog Update Success
-
Image Cacheing with Glide (Preloader)
-
Begin Part 5
-
Part 5 Introduction
-
CreateBlog: ViewModel, State and Repository
-
Selecting and Cropping an Image
-
Uploading Image to Server with Retrofit
-
Updating a Blog Post
-
Part 6: Refactoring
-
Dagger Refactor
-
Process Death Issue Fix (Part 1/2)
-
Process Death Issue Fix (Part 2/2)
-
BackStack Bug
-
Navigation Bug
-
Remove dagger-android
-
Flows and Channels Refactor